How to Convert a C++ String to Uppercase
Solution 1
You need to put a double colon before toupper
:
transform(input.begin(), input.end(), input.begin(), ::toupper);
Explanation:
There are two different toupper
functions:
toupper
in the global namespace (accessed with::toupper
), which comes from C.toupper
in thestd
namespace (accessed withstd::toupper
) which has multiple overloads and thus cannot be simply referenced with a name only. You have to explicitly cast it to a specific function signature in order to be referenced, but the code for getting a function pointer looks ugly:static_cast<int (*)(int)>(&std::toupper)
Since you're using namespace std
, when writing toupper
, 2. hides 1. and is thus chosen, according to name resolution rules.
Solution 2
Boost string algorithms:
#include <boost/algorithm/string.hpp>
#include <string>
std::string str = "Hello World";
boost::to_upper(str);
std::string newstr = boost::to_upper_copy("Hello World");
Convert a String In C++ To Upper Case
Solution 3
Try this small program, straight from C++ reference
#include <iostream>
#include <algorithm>
#include <string>
#include <functional>
#include <cctype>
using namespace std;
int main()
{
string s;
cin >> s;
std::transform(s.begin(), s.end(), s.begin(), std::ptr_fun<int, int>(std::toupper));
cout << s;
return 0;
}
Solution 4
You could do:
string name = "john doe"; //or just get string from user...
for(int i = 0; i < name.size(); i++) {
name.at(i) = toupper(name.at(i));
}
Solution 5
#include <iostream>
using namespace std;
//function for converting string to upper
string stringToUpper(string oString){
for(int i = 0; i < oString.length(); i++){
oString[i] = toupper(oString[i]);
}
return oString;
}
int main()
{
//use the function to convert string. No additional variables needed.
cout << stringToUpper("Hello world!") << endl;
return 0;
}
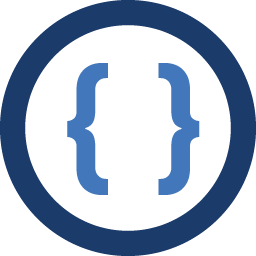
Admin
Updated on May 31, 2021Comments
-
Admin almost 3 years
I need to convert a string in C++ to full upper case. I've been searching for a while and found one way to do it:
#include <iostream> #include <algorithm> #include <string> using namespace std; int main() { string input; cin >> input; transform(input.begin(), input.end(), input.begin(), toupper); cout << input; return 0; }
Unfortunately this did not work and I received this error message:
no matching function for call to 'transform(std::basic_string::iterator, std::basic_string::iterator, std::basic_string::iterator,
I've tried other methods that also did not work. This was the closest to working.
So what I'm asking is what I am doing wrong. Maybe my syntax is bad or I need to include something. I am not sure.
I got most of my info here: http://www.cplusplus.com/forum/beginner/75634/ (last two posts)