How to convert a Java 8 Stream to an Array?
Solution 1
The easiest method is to use the toArray(IntFunction<A[]> generator)
method with an array constructor reference. This is suggested in the API documentation for the method.
String[] stringArray = stringStream.toArray(String[]::new);
What it does is find a method that takes in an integer (the size) as argument, and returns a String[]
, which is exactly what (one of the overloads of) new String[]
does.
You could also write your own IntFunction
:
Stream<String> stringStream = ...;
String[] stringArray = stringStream.toArray(size -> new String[size]);
The purpose of the IntFunction<A[]> generator
is to convert an integer, the size of the array, to a new array.
Example code:
Stream<String> stringStream = Stream.of("a", "b", "c");
String[] stringArray = stringStream.toArray(size -> new String[size]);
Arrays.stream(stringArray).forEach(System.out::println);
Prints:
a
b
c
Solution 2
If you want to get an array of ints, with values from 1 to 10, from a Stream<Integer>
, there is IntStream
at your disposal.
Here we create a Stream
with a Stream.of
method and convert a Stream<Integer>
to an IntStream
using a mapToInt
. Then we can call IntStream
's toArray
method.
Stream<Integer> stream = Stream.of(1,2,3,4,5,6,7,8,9,10);
//or use this to create our stream
//Stream<Integer> stream = IntStream.rangeClosed(1, 10).boxed();
int[] array = stream.mapToInt(x -> x).toArray();
Here is the same thing, without the Stream<Integer>
, using only the IntStream
:
int[]array2 = IntStream.rangeClosed(1, 10).toArray();
Solution 3
You can convert a java 8 stream to an array using this simple code block:
String[] myNewArray3 = myNewStream.toArray(String[]::new);
But let's explain things more, first, let's Create a list of string filled with three values:
String[] stringList = {"Bachiri","Taoufiq","Abderrahman"};
Create a stream from the given Array :
Stream<String> stringStream = Arrays.stream(stringList);
we can now perform some operations on this stream Ex:
Stream<String> myNewStream = stringStream.map(s -> s.toUpperCase());
and finally convert it to a java 8 Array using these methods:
1-Classic method (Functional interface)
IntFunction<String[]> intFunction = new IntFunction<String[]>() {
@Override
public String[] apply(int value) {
return new String[value];
}
};
String[] myNewArray = myNewStream.toArray(intFunction);
2 -Lambda expression
String[] myNewArray2 = myNewStream.toArray(value -> new String[value]);
3- Method reference
String[] myNewArray3 = myNewStream.toArray(String[]::new);
Method reference Explanation:
It's another way of writing a lambda expression that it's strictly equivalent to the other.
Solution 4
Convert text to string array where separating each value by comma, and trim every field, for example:
String[] stringArray = Arrays.stream(line.split(",")).map(String::trim).toArray(String[]::new);
Solution 5
import java.util.List;
import java.util.stream.Stream;
class Main {
public static void main(String[] args) {
// Create a stream of strings from list of strings
Stream<String> myStreamOfStrings = List.of("lala", "foo", "bar").stream();
// Convert stream to array by using toArray method
String[] myArrayOfStrings = myStreamOfStrings.toArray(String[]::new);
// Print results
for (String string : myArrayOfStrings) {
System.out.println(string);
}
}
}
Try it out online: https://repl.it/@SmaMa/Stream-to-array
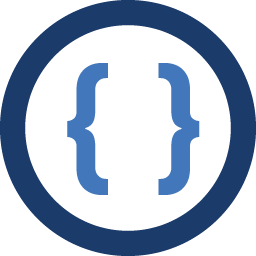
Admin
Updated on December 04, 2020Comments
-
Admin over 3 years
What is the easiest/shortest way to convert a Java 8
Stream
into an array? -
jarek.jpa over 7 yearsand here is an explanation why and how the Array constructor reference actually work: stackoverflow.com/questions/29447561/…
-
scottb about 7 years"Zenexer is right, the solution should be: stream.toArray(String[]::new);" ... Well ok, but one should understand that the method reference is logically and functionally equivalent to
toArray(sz -> new String[sz])
so I'm not sure that one can really say what the solution should or must be. -
Andy Thomas about 7 yearsThe array constructor reference is more concise. The documentation for the
toArray()
method cites its concision and includes its use in the only example. When it was tacked on the end of the answer, I missed it on first reading. Moved it to the top, above the details, so that no one else will miss it. -
WORMSS over 6 years@scottb
sz -> new String[sz]
makes a new function where as the constructor reference does not. It depends how much you value Garbage Collection Churn I guess. -
HTNW over 6 years@WORMSS It does not. It (statically!) makes a new,
private
method, which cannot cause churn, and both versions need to create a new object. A reference creates an object that points directly at the target method; a lambda creates an object that points at the generatedprivate
one. A reference to a constructor should still perform better for lack of indirection and easier VM optimization, but churning has nothing to do with it. -
WORMSS over 6 years@HTNW you are correct, my apologise. It was infact my attempt to debug that was causing the churn that was causing the churn the first time I tried to do this, so I have had it stuck in my head that this is how it was. (Hate it when that happens).
-
Didier L almost 6 yearsWhy would you use this instead of Stream.toArray(IntFunction)?
-
Ole V.V. over 5 yearsI needed a collector to pass to the 2-arg
Collectors.groupingBy
so that I could map some attribute to arrays of objects per attribute value. This answer gives me exactly that. Also @DidierL. -
curiouscupcake about 4 yearsWhat is the difference between your answer and the accepted answer?
-
Sma Ma about 4 years@LongNguyen It's a full example including an online replay scenario, not only a snippet.
-
bjmi over 3 yearsSince Java 11 the finisher in collectingAndThen can be written as
list -> list.toArray(converter)
due to addition of Collection.toArray(IntFunction) -
user1169587 over 3 years"String[] stringArray = stringStream.toArray(size -> new String[size]);", where is the size come from?
-
Steven Shi about 3 yearsQuestion is [] not ArrayList
-
djmj about 3 yearsLambda should simplify things but java lambda like
stream.toArray(size -> new String[size]);
just looks akward and this is so unintuitive every developer will forget this syntax the next time he needs an array. Why do they make things so complicated where c# is making life pretty easy with lambda. My stack is java but since years java getting more and more behind of c#. -
Matt almost 2 yearsInstead of
stream.toArray(size -> new String[size])
, you can shorten it using a method reference:stream.toArray(String[]::new)
, which accomplishes the same thing. Syntactic sugar, if you have a sweet tooth.