How to convert a string to a complex number in Python?
Solution 1
From the documentation:
Note
When converting from a string, the string must not contain whitespace around the central + or - operator. For example,
complex('1+2j')
is fine, butcomplex('1 + 2j')
raisesValueError
.
Solution 2
Following the answer from Francisco Couzo, the documentation states that
When converting from a string, the string must not contain whitespace around the central + or - operator. For example, complex('1+2j') is fine, but complex('1 + 2j') raises ValueError.
Remove all the spaces from the string and you'll get it done, this code works for me:
a = "3 + 3j"
a = a.replace(" ", "") # will do nothing if unneeded
b = complex(a)
Solution 3
complex
's constructor rejects embedded whitespace. Remove it, and it will work just fine:
>>> complex(''.join(a.split())) # Remove all whitespace first
(3+3j)
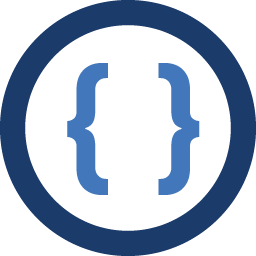
Admin
Updated on July 27, 2022Comments
-
Admin almost 2 years
I'm trying to convert an input string to a float but when I do it I keep getting some kind of error, as shown in the sample below.
>>> a = "3 + 3j" >>> b = complex(a) Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: complex() arg is a malformed string
-
Tyberius about 2 yearsEval you have to careful once you move to the case of parsing text from user input or a file rather than just entering your own complex number as a string.