how to convert binary to decimal using python without using the bin function
18,030
Solution 1
To convert from binary to decimal, just do:
int(binary_string, 2) # The 2 is the base argument from which we convert.
Demo:
>>> int('0b10110', 2)
22
Note- There are many issues with the code you are using to convert decimal to binary. If you are insistent on not using a built-in function for that purpose, you may be interested by this post:
Convert an integer to binary without using the built-in bin function
Although personally if I were to want to avoid the bin()
function, I would do something like:
"{0:#b}".format(an_integer)
Demo:
>>> "{0:#b}".format(22)
'0b10110'
This is much more Pythonic than your current code.
Solution 2
this would be a lot easier no?
conv = str(input('what do you want to convert?'))
if conv == 'binary':
x = str(input('enter binary : '))
ansBin = int(x, 2)
print('decimal for binary ', x, ' is ', ansBin)
elif conv == 'decimal':
y = int(input('enter decimal : '))
ansDec = bin(y)[2:]
print('binary for decimal ', y, ' is ', ansDec)
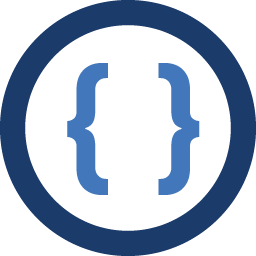
Author by
Admin
Updated on July 29, 2022Comments
-
Admin over 1 year
iv made a decimal to binary converter but I need a binary to decimal converter without using the bin function and that looks simple enough to fit with this code. can anyone help?
choice=input('Please enter b for a binary to decimal conversion or d for a decimal to binary conversion:\n') if choice == 'd': decimalNum=int(input('Please enter a decimal number:\n')) bit8=0 bit7=0 bit6=0 bit5=0 bit4=0 bit3=0 bit2=0 bit1=0 bit8=decimalNum%2 decimalNum=decimalNum//2 bit7=decimalNum%2 decimalNum=decimalNum//2 bit6=decimalNum%2 decimalNum=decimalNum//2 bit5=decimalNum%2 decimalNum=decimalNum//2 bit4=decimalNum%2 decimalNum=decimalNum//2 bit3=decimalNum%2 decimalNum=decimalNum//2 bit2=decimalNum%2 decimalNum=decimalNum//2 bit1=decimalNum%2 decimalNum=decimalNum//2 print(str(bit1)+str(bit2)+str(bit3)+str(bit4)+str(bit5)+str(bit6)+str(bit7)+str(bit7))
-
SuperBiasedMan over 8 yearsPlease consider editing your post to add more explanation about what your code does and why it will solve the problem. An answer that mostly just contains code (even if it's working) usually wont help the OP to understand their problem. It's also recommended that you don't post an answer if it's just a guess. A good answer will have a plausible reason for why it could solve the OP's issue.