How to convert list of Objects to JSON in Spring MVC and Hibernate?
17,019
You need to assign returned json value to String
object and then pass it to ModelAndView
. Refer Gson.toJson()
and also make sure your class(EmployeeBean
) is serializable
.
@RequestMapping(value = "/employees1", method = RequestMethod.GET)
public ModelAndView employeelist() {
Gson gson = new Gson();
String json = gson.toJson(employeeService.listEmployeess());
ModelAndView modelAndView = new ModelAndView();
modelAndView.addObject(json);
return modelAndView;
}
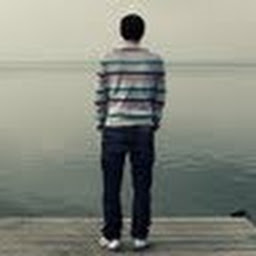
Author by
Nikhil Bharadwaj
Updated on June 04, 2022Comments
-
Nikhil Bharadwaj almost 2 years
I'm Using Spring MVC and hibernate to fetch data from MySQL. In the controller class a ModelAndView method listEmployees is returning a MAP.The method is getting list of Employee objects from EmployeeService class. I want this method to take list of Employee Objects from Service class and covert it into JSON,or convert it into JSON and write that data in a JSON file so that I can use this JSON data in a JSP page.
@RequestMapping(value="/employees", method = RequestMethod.GET) public @ResponseBody ModelAndView listEmployees() { Map<String, Object> model = new HashMap<String, Object>(); model.put("employees",prepareListofBean(employeeService.listEmployeess())); return new ModelAndView("employeesList", model); } private List<EmployeeBean> prepareListofBean(List<Employee> employees){ List<EmployeeBean> beans = null; if(employees != null && !employees.isEmpty()){ beans = new ArrayList<EmployeeBean>(); EmployeeBean bean = null; for(Employee employee : employees){ bean = new EmployeeBean(); bean.setName(employee.getEmpName()); bean.setId(employee.getEmpId()); bean.setAddress(employee.getEmpAddress()); bean.setSalary(employee.getSalary()); bean.setAge(employee.getEmpAge()); beans.add(bean); } } return beans; }
EDIT
In this below method I tried GSON to convert list to JSON,but was getting null pointer Exception.
**@RequestMapping(value="/employees1", method = RequestMethod.GET) public ModelAndView employeelist() { Gson gson=new Gson(); gson.toJson(employeeService.listEmployeess()); ModelAndView modelAndView=new ModelAndView(); modelAndView.addObject(gson); return modelAndView; }**
What could be the mistake in this above method?
-
Naman Gala over 8 years
I can use this JSON data in a JSP page
, how you want to use it? Using ajax call? -
Raman Shrivastava over 8 yearsThere are many libraries built around this e.g. Jackson, GSON etc. Check those. Look for basic tutorials on them. And try posting your attempt rather than asking blind question.
-
Nikhil Bharadwaj over 8 years@Raman Shrivastava,please see my edit.
-
Raman Shrivastava over 8 yearslistEmployeess() - spelling is wrong based upon your method declaration in initial code. toJson() returns a String. Why are you not storing toJson()'s result in a String?
-
-
Nikhil Bharadwaj over 8 years,Thanks.That helped a lot.