How to convert Local to UTC and UTC to Local in angular5?
Solution 1
You could use the moment module in angular.
import * as moment from "moment";
From local to UTC:
moment(local_date).toDate();
From UTC to local:
moment.utc(utc_date).toDate()
Solution 2
One approach could be to only convert to UTC once, after the user enters their time. Then store the UTC value in your database. When you want to display the dateTime again to the client you can utilize Angulars built in Pipe, DatePipe , to manage the logic for converting from UTC to your desired format.
In your component get the UTC date from your server:
import {Component, OnInit} from '@angular/core';
@Component({
selector: 'input-overview-example',
templateUrl: 'date-example.html',
})
export class DateExample implements OnInit {
public utcDate;
ngOnInit(){
//get the UTC date from your server
this.utcDate = new Date('2016-11-07T22:46:47.267');
}
}
Then use the DatePipe to convert the UTC in your template to your desired format:
<h3>Example UTC Date pipe conversion</h3>
<div>{{utcDate| date:"MM/dd/yy"}}</div>
Check out the StackBlitz demo I made if you want to play around with it: Live demo
Solution 3
Do not add/subtract an offset. That is just picking a different moment in time. Instead, simply call .toISOString()
to generate the UTC-based ISO8601-formatted string to send to your server. You can also pass such string into the Date
object's constructor. All conversion to local time will happen when you format the Date
object into a string.
Solution 4
in ts
import { Component, OnInit } from '@angular/core';
import { utc } from 'moment';
@Component({
selector: 'input-overview-example',
templateUrl: 'date-example.html'
})
export class DateExample implements OnInit {
utcDateStr: string;
utc = utc;
ngOnInit() {
this.utcDateStr = '2016-11-07T22:46:47.267';
}
}
in html
{{ utc(utcDateStr).local().format("DD/MM/YYYY HH:MM")}}
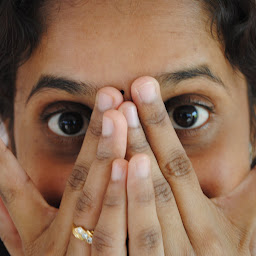
Neelima Ediga
Experienced Senior Technical Engineer with a demonstrated history of working in the Information Technology and Services Industry. Skilled in ASP.Net, C#, SQL Server Database, and other advanced technologies.
Updated on June 05, 2022Comments
-
Neelima Ediga almost 2 years
I have a DateTimePicker on my page, where the user selects the datetime in their local timezone. But when we save that in the DB, I need to convert this to UTC and save it. Similarly, while displaying this to the user, again I need to convert from UTC to user's local timezone.
Saving: LOCAL timezone to UTC
Displaying: UTC to LOCAL timezoneAs the server might reside on other locations, I thought it would be correct to convert the value to UTC at the client itself. Not sure, if there is a better way of doing this. So, was trying for the same in my angular5 page.
Not so familiar with moment.js, and so couldn't completely understand on how it actually works. Any tips to do this in a better way in angular?
Below is how I achieved..
convertUtcToLocalString(val : Date) : string { var d = new Date(val); // val is in UTC var localOffset = d.getTimezoneOffset() * 60000; var localTime = d.getTime() - localOffset; d.setTime(localTime); return this.formatDate(d); } convertUtcToLocalDate(val : Date) : Date { var d = new Date(val); // val is in UTC var localOffset = d.getTimezoneOffset() * 60000; var localTime = d.getTime() - localOffset; d.setTime(localTime); return d; } convertLocalToUtc(val : Date) : Date { var d = new Date(val); var localOffset = d.getTimezoneOffset() * 60000; var utcTime = d.getTime() + localOffset; d.setTime(utcTime); return d; }
Appreciate your help.
Thanks!
-
Neelima Ediga about 6 yearsThanks for your reply. While saving it, I was able to convert and save it in UTC. However, couldn't convert to local timezone while displaying it. Do you mind letting me know on how this DatePipe can be used for this?
-
Narm about 6 yearsSure, could you show how you are trying to use the pipe in your template code and what your UTC date looks like?
-
Neelima Ediga about 6 yearsI added the piece of code in the below, as I couldnt format it properly here. Many thanks again for your response.
-
Neelima Ediga about 6 yearsNot sure if I get it completely. But I do not have an option to save these details in the server. And so was trying to handle it at the client side. Thank you
-
Narm about 6 years@NeelimaEdiga, updated my answer. Hope that helps! :)
-
Matt Johnson-Pint about 6 yearsThe client-side JavaScript
Date
object will do all this for you. You are fighting against it. Read more about theDate
object and how it works, and you'll understand.