how to convert QString into int in qt creator
10,398
Solution 1
You need to get rid of the bitrate:
prefix and kb/s
suffix. The best way is to use QRegExp
or QRegularExpression
to extract the digit part of the string and then call toInt()
.
Here's example:
QString str = "bitrate: 3543 kb/s";
int value;
QRegExp re("bitrate:\\s*(\\d+)\\s*.*");
if (re.indexIn(str) != -1) {
value = re.cap(1).toInt();
} else {
qDebug() << "String not matched.";
return;
}
qDebug() << value;
Solution 2
The reference page has a ton of material on this. The easiest way to implement this is to split the string based on spaces, then convert the one you want to an Int.
Example:
QString str = "bitrate: 3543 kb/s";
QStringList lst = str.split(" ");
Int value = lst[1].toInt();
Solution 3
QTextIStream stream("bitrate: 3543 kb/s");
QString prefix, sufix;
int result;
stream >> prefix >> result >> sufix;
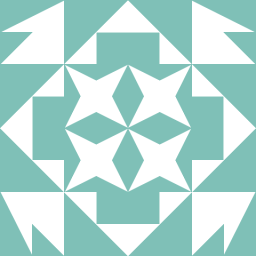
Author by
Mlarnt90
Updated on June 14, 2022Comments
-
Mlarnt90 almost 2 years
I have seen one answer regarding to this same question but when I tried it with my problem it get confused.
here is my string,
bit_rate = "bitrate: 2334 kb/s"
I need to get the
2334
from this string and assign it into a integer variable.. how is this possible in qt creator. I triedtoInt()
, but it always gives0
as the answer. -
Mlarnt90 almost 10 yearsthanx that exactly gave me the answer I wanted.
-
Mlarnt90 almost 10 yearsoops I was being silly. thanx ur answer gave me the result very easily..thank you very much.
-
Eitan T almost 10 years@Mlarnt90 so the answer to duplicate question didn't help you? It's basically the same as this one.
-
Mlarnt90 almost 10 yearsthanx @Googie for your answer. Because of it, I tend to use regular expressions for other parts in my code.