How to copy file using Rest API and javascript in Sharepoint 2013 between site and subsite
Solution 1
Just figured this one out today for the cross site solution. The trick is-- don't use $.ajax for the download of the document. Use good old XMLHttpRequest. The reason is that JQuery simply doesn't let you get a raw binary data array from SharePoint. But, the XMLHttpRequest does because it allows you to get an arraybuffer as part of its implementation, which SharePoint accepts!
The following is the code with the parts identified for building the full source and target REST urls. Note that you can use $.ajax to upload the file.
- sourceSite is a sharepoint site suitable for appending the '_api' rest endpoint
- sourceFolderPath is the relative folder path your document is located within
- sourceFileName is the filename of the document
- targetSite, targetFolderPath and targetFileName are the mirror images or source, only for the destination.
-
requestDigest is that special value you need for SharePoint to accept updates.
function copyDocument(sourceSite, sourceFolderPath, sourceFileName, targetSite, targetFolderPath, targetFileName, requestDigest) { var sourceSiteUrl = sourceSite + "_api/web/GetFolderByServerRelativeUrl('" + sourceFolderPath + "')/Files('" + sourceFileName + "')/$value"; var targetSiteUrl = targetSite + "_api/web/GetFolderByServerRelativeUrl('" + targetFolderPath + "')/Files/Add(url='" + targetFileName + "',overwrite=true)"; var xhr = new XMLHttpRequest(); xhr.open('GET', sourceSiteUrl, true); xhr.setRequestHeader('binaryStringResponseBody', true); xhr.responseType = 'arraybuffer'; xhr.onload = function (e) { if (this.status == 200) { var arrayBuffer = this.response; $.ajax({ url: targetSiteUrl, method: 'POST', data: arrayBuffer, processData: false, headers: { 'binaryStringRequestBody': 'true', 'Accept': 'application/json;odata=verbose;charset=utf-8', 'X-RequestDigest': requestDigest } }) .done(function (postData) { console.log('we did it!'); }) .fail(function (jqXHR, errorText) { console.log('dadgummit'); }); } } xhr.send(); }
Solution 2
What kind of error are you getting?
One probable cause of your problem is that your RequestDigest does not match the location where you want to POST your file since it is fetched from the page where your code is running. Fetch a matching RequestDigest by calling '_api/contextinfo' on your target location.
See: http://blogs.breeze.net/mickb/2012/11/20/SP2013GettingAFormDigestForUpdateRESTCalls.aspx and http://msdn.microsoft.com/en-us/magazine/dn198245.aspx (writing to Sharepoint section)
Solution 3
Note File Move operations only work within the scope of a given document library. You cannot copy between document libraries.
http://msdn.microsoft.com/en-us/library/office/dn605900(v=office.15).aspx#Folder6
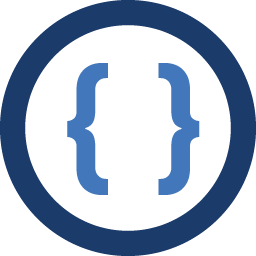
Admin
Updated on June 29, 2022Comments
-
Admin almost 2 years
I need to copy file between document libraries. Library A is located in one site and Library B is located in subsite. I know how to copy file between libraries on the same level but the problem is with copying between different level.
The code I use to copy file between libraries on the same level.
$.ajax({ url : "http://xxx/PWA/_api/web/folders/GetByUrl('/PWA/CopyFromLibrary')/Files/getbyurl('Import.csv')/copyTo(strNewUrl = '/PWA/TargetLibrary/Import.csv',bOverWrite = true)", method: 'POST', headers: { "Accept": "application/json; odata=verbose", "X-RequestDigest": $("#__REQUESTDIGEST").val() }, success: function () { alert("Success! Your file was copied properly"); }, error: function () { alert("Problem with copying"); } });
For different level I use just another target URL:
url : "http://xxx/PWA/_api/web/folders/GetByUrl('/PWA/CopyFromLibrary')/Files/getbyurl('Import.csv')/copyTo(strNewUrl = '/PWA/Subsite/TargetLibrary/Import.csv',bOverWrite = true)",
And it doesn't work. How to work around this problem?
-
Adi over 7 yearsThanks it Worked!!! :) . I was searching for it from Long Time :) .1 more thing if requestDigest value would be $("#__REQUESTDIGEST").val()
-
Mark over 4 yearsWhat should be the syntax to get requestDigest with right value?
-
Manveer Singh over 3 yearsIt's not coping all properties of document, is there any fix for it?