How to correctly initialize a class
Looks like the exercise underwent multiple variable updates and they forgot to update the template for a lot of the init values. Anyways this should work fine.
main.py
from Artist import Artist
from Artwork import Artwork
if __name__ == "__main__":
user_artist_name = input()
user_birth_year = int(input())
user_death_year = int(input())
user_title = input()
user_year_created = int(input())
user_artist = Artist(user_artist_name, user_birth_year, user_death_year)
new_artwork = Artwork(user_title, user_year_created, user_artist)
new_artwork.print_info()
Artist.py
class Artist:
def __init__(self, name="None", birth_year=0, death_year=0):
self.name = name
self.birth_year = birth_year
self.death_year = death_year
def print_info(self):
if self.death_year == -1:
print('Artist: {}, born {}'.format(self.name, self.birth_year))
else:
print('Artist: {} ({}-{})'.format(self.name, self.birth_year, self.death_year))
Artwork.py
from Artist import Artist
class Artwork:
def __init__(self, user_title="None", year_created=0, user_artist=Artist()):
self.title = user_title
self.year_created = year_created
self.artist = user_artist
def print_info(self):
self.artist.print_info()
print('Title: %s, %d' % (self.title, self.year_created))
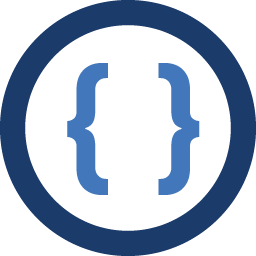
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
For my python assignment, I have to create classes and initialize them. I created them correctly but the automatic grader says they are not correctly initialized.
Define the Artist class with a constructor to initialize an artist's information and a print_info() method. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0. print_info() should display Artist Name, born XXXX if the year of death is -1 or Artist Name (XXXX-YYYY) otherwise.
Define the Artwork class with a constructor to initialize an artwork's information and a print_info() method. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values.
What I have so far:
class Artist: def __init__(self, user_artist_name="None", user_birth_year=0, user_death_year=0): self.name = user_artist_name self.birth = user_birth_year self.death = user_death_year def print_info(self): if self.death == -1: print("Artist: {}, born {}".format(self.name, self.birth)) else: print("Artist: {} ({}-{})".format(self.name, self.birth, self.death)) class Artwork: def __init__(self, user_title= "None", year_created=0, user_artist=Artist()): self.title = user_title self.year = year_created self.artist = user_artist def print_info(self): print("Title: {}, {}".format(self.title, self.year)) if __name__ == "__main__": user_artist_name = input() user_birth_year = int(input()) user_death_year = int(input()) user_title = input() user_year_created = int(input()) user_artist = Artist(user_artist_name, user_birth_year, user_death_year) user_artist.print_info() new_artwork = Artwork(user_title, user_year_created, user_artist) new_artwork.print_info()
Artist('Pablo Picasso', 1881, 1973) fails to correctly initialize artist. and the constructor for both default parameters of Artist and Artwork fail.
What am I missing?
-
Pranav Hosangadi over 3 yearsYour code looks perfectly fine! Get in touch with the prof. Maybe they meant "
print_info()
should display Artist Name, born XXXX if the year of death is 0" -
Barmar over 3 yearsI tried
a = Artist('Pablo Picasso', 1881, 1973)
thena.print_info()
and it worked perfectly. Why do you think it doesn't work? -
Barmar over 3 yearsDon't use the default value
user_artist = Artist()
. See stackoverflow.com/questions/1132941/… -
Prune over 3 yearsPlease supply the expected minimal, reproducible example. Show where the intermediate results differ from what you expected. We should be able to copy and paste a contiguous block of your code, execute that file, and reproduce your problem along with tracing output for the problem points. This lets us test our suggestions against your test data and desired output.
-
Prune over 3 yearsDon't expect us to guess what your auto-grader wants; give us a test case that fails, along with the results. If you have trouble matching your assignment statement to a black-box grader that we can't even access, then you should take up the problem with your instructor or classmates.
-
Admin over 3 years@Prune the code above is the test case that failed. imgur.com/Vc4CFTX this is the result of me entering that code in. It automatically enters in : Pablo Picasso 1881 1973 Three Musicians 1921
-
Pranav Hosangadi over 3 yearsCould they want you to initialize the names to
None
instead of"None"
? IDK why they'd say"None"
then, but the answer to your question is ask your prof. or TA. We don't know what they intended for their unit tests to check, and it seems like your code fulfils all requirements specified in the problem description. -
Prune over 3 yearsPlease refer to the posting guidelines and complete the posting: your post must be self-contained. Off-site links are not acceptable. You have several people trying to guess as to your problem requirements. That may be appropriate for a help site, but does not fit Stack Overflow.
-