How to create a custom packet in c?
Solution 1
A relatively easy tool to do this that is portable is libpcap
. It's better known for receiving raw packets (and indeed it's better you play with that first as you can compare received packets with your hand crafted ones) but the little known pcap_sendpacket
will actually send a raw packet.
If you want to do it from scratch yourself, open a socket with AF_PACKET
and SOCK_RAW
(that's for Linux, other OS's may vary) - for example see http://austinmarton.wordpress.com/2011/09/14/sending-raw-ethernet-packets-from-a-specific-interface-in-c-on-linux/ and the full code at https://gist.github.com/austinmarton/1922600 . Note you need to be root (or more accurately have the appropriate capability) to do this.
Also note that if you are trying to send raw tcp/udp packets, one problem you will have is disabling the network stack automatically processing the reply (either by treating it as addressed to an existing IP address or attempting to forward it).
Solution 2
Doing this sort of this is not as simple as you think. Controlling the data above the IP layer is relatively easy using normal socket APIs, but controlling data below is a bit more involved. Most operating systems make changing lower-level protocol information difficult since the kernel itself manages network connections and doesn't want you messing things up. Beyond that, there are other platform differences, network controls, etc that can play havoc on you.
You should look into some of the libraries that are out there to do this. Some examples:
- libnet - http://libnet.sourceforge.net/
- libdnet - http://libdnet.sourceforge.net/
If your goal is to spoof packets, you should read up on network-based spoofing mitigation techniques too (for example egress filtering to prevent spoofed packets from exiting a network).
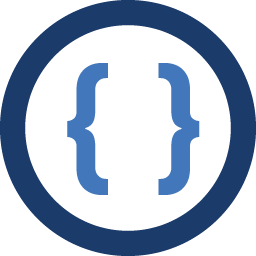
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I'm trying to make a custom packet using C using the TCP/IP protocol. When I say custom, I mean being able to change any value from the packet; ex: MAC, IP address and so on.
I tried searching around but I can't find anything that is actually guiding me or giving me example source codes.
How can I create a custom packet or where should I look for guidance?
-
Admin about 10 yearsBelieve me I was prepared for it to be pretty complicated and thanks for the libraries but do you have anything else then libraries?
-
Admin about 10 yearsI'm on Linux and have root access, but how do you disable the network stack automatically processing the reply or make the packet skip it so it doesn't have to get its IP changed?
-
abligh about 10 yearsThat's non-trivial. Probably the easiest route is to use an ip address not assigned to the system, and either do your own
arp
or set a manual arp entry. You may need to turn on ip forwarding then askiptables
to drop packets (libpcap
should still pick them up) in theFORWARD
chain to prevent it sending ICMP host unreachable. -
abligh about 10 yearsIf you absolutely must use the same IP address as the server's OS, the issue is finding which TCP ports are free - essentially you want two TCP stacks sharing the same IP. I've done this once by using NAT and a tunnel interface, so my connection comes from the remote end of a tunnel (i.e. user space) then masquerades (using NAT) as the real IP address. This is fiddly to get right.
-
Admin about 10 yearsTrue, but what if I wanted to insert a code were the sender's ip is in the packet, how do I do that?
-
abligh about 10 yearsYou can send it out, but what the OS will do with the reply packet is the problem. See my second comment for how I got around this. If you are not using a protocol the OS is using or can persuade
iptables
to drop these packets on itsINPUT
chain, you won't have an issue. -
Admin about 10 yearsI'm intending to actually put the receiver's IP has the receiver and sender's IP in the packet. Well use TCP has the protocol, wich is used by both the sender and receiver's OS. But will the OS try to block or stop this?
-
abligh about 10 yearsYes it will (unless you stop it), because either the OS will see it as a TCP session it doesn't recognise (and probably send an
RST
), or it will see it as a TCP session it does recognise (as you have reused existing port tuples), in which case your sequence number will inevitably be wrong; what it does there 'depends' - probably ignores it. -
Admin about 10 years,thanks for the tips bro, but how do you get to send it and that your OS doesn't stop it or modifies it?Is it possible in c or do I have go into assembler?
-
abligh about 10 yearsAssembler won't help you. I think I've already told you how to do this. Persuade the OS to drop the incoming packet (you will still pick it up with
libpcap
) usingiptables
. I've told you how I used an entirely different technique and persuaded my app and the OS to share TCP port space using a tunnel (tun
device) and usingiptables
to masquerade routed packets from the tunnel as the source IP of the box; in this method you read and write bytes from an FD which are the raw bytes of the IP packet. You write your packets as if they come from a dummy IP and the OS rewrites the source addr. -
jduck about 10 yearsThere are a lot of moving parts in doing this. The reason I recommend these libraries is because they deal with many of them for you. If you're interested in how they work, I recommend reading the source code.