How to create a different ThreadPoolTaskExecutor in Spring Boot?
17,750
Solution 1
First of all, you can define your thread pool executor and use them as a configure them as beans like this -
@Configuration
public class ThreadConfig {
@Bean
public TaskExecutor executorA() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(4);
executor.setMaxPoolSize(4);
executor.setThreadNamePrefix("default_task_executor_thread");
executor.initialize();
return executor;
}
@Bean
public TaskExecutor executorB() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(4);
executor.setMaxPoolSize(4);
executor.setThreadNamePrefix("executor-B");
executor.initialize();
return executor;
}
}
After that, you can specify your executor on method level like this -
@Async("executorA")
public void methodWithVoidReturnType(String s) {
.....
}
@Async("executorA")
public Future<String> methodWithSomeReturnType() {
...
try {
Thread.sleep(5000);
return new AsyncResult<String>("hello world !!!!");
} catch (InterruptedException e) {
...
}
return null;
}
Solution 2
@Bean(name = "threadPoolExecutor")
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(7);
executor.setMaxPoolSize(42);
executor.setQueueCapacity(11);
executor.setThreadNamePrefix("threadPoolExecutor-");
executor.initialize();
return executor;
}
@Bean(name = "ConcurrentTaskExecutor")
public TaskExecutor taskExecutor2 () {
return new ConcurrentTaskExecutor(
Executors.newFixedThreadPool(3));
}
@Override
@Async("threadPoolExecutor")
public void createUserWithThreadPoolExecutor(){
System.out.println("Currently Executing thread name - " + Thread.currentThread().getName());
System.out.println("User created with thread pool executor");
}
@Override
@Async("ConcurrentTaskExecutor")
public void createUserWithConcurrentExecutor(){
System.out.println("Currently Executing thread name - " + Thread.currentThread().getName());
System.out.println("User created with concurrent task executor");
}
Related videos on Youtube
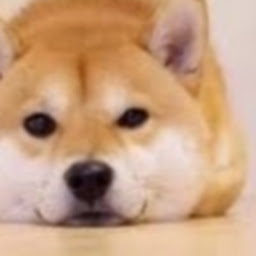
Author by
DD Jin
Updated on July 18, 2022Comments
-
DD Jin almost 2 years
I am now using
@EnableAsync
and@Async
annotation to use multithreaded in Spring Boot. I have service A(fast) and service B(slow).How can I set different pool for them? So when there are lots of calls for B, the application can still handle service A in a different pool from B.
@Configuration @EnableAsync public class ServiceExecutorConfig implements AsyncConfigurer { @Override public Executor getAsyncExecutor() { ThreadPoolTaskExecutor taskExecutor = new ThreadPoolTaskExecutor(); taskExecutor.setCorePoolSize(30); taskExecutor.setMaxPoolSize(40); taskExecutor.setQueueCapacity(10); taskExecutor.initialize(); return taskExecutor; } }
-
Rangad over 4 yearsMaybe this might help you: How to use multiple threadPoolExecutor for Async Spring
-
-
DD Jin over 4 yearsIn this way how can I set the AsyncUncaughtExceptionHandler?