How to create a folder in Java?
18,631
Solution 1
File f = new File("C:\\TEST");
try{
if(f.mkdir()) {
System.out.println("Directory Created");
} else {
System.out.println("Directory is not created");
}
} catch(Exception e){
e.printStackTrace();
}
Solution 2
Call File.mkdir
, like this:
new File(path).mkdir();
Solution 3
With Java 7 and newer you can use the static Files.createDirectory() method of the java.nio.file.Files
class along with Paths.get
.
Files.createDirectory(Paths.get("/path/to/folder"));
The method Files.createDirectories() also creates parent directories if these do not exist.
Solution 4
Use mkdir()
:
new File('/path/to/folder').mkdir();
Solution 5
Use the mkdir method on the File class:
https://docs.oracle.com/javase/1.5.0/docs/api/java/io/File.html#mkdir%28%29
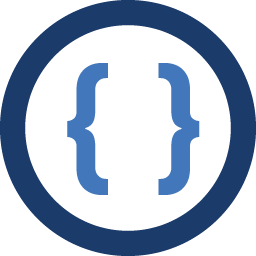
Author by
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
How can I create an empty folder in Java?