How to create a template function within a class? (C++)
Solution 1
Your guess is the correct one. The only thing you have to remember is that the member function template definition (in addition to the declaration) should be in the header file, not the cpp, though it does not have to be in the body of the class declaration itself.
Solution 2
See here: Templates, template methods,Member Templates, Member Function Templates
class Vector
{
int array[3];
template <class TVECTOR2>
void eqAdd(TVECTOR2 v2);
};
template <class TVECTOR2>
void Vector::eqAdd(TVECTOR2 a2)
{
for (int i(0); i < 3; ++i) array[i] += a2[i];
}
Solution 3
Yes, template member functions are perfectly legal and useful on numerous occasions.
The only caveat is that template member functions cannot be virtual.
Solution 4
The easiest way is to put the declaration and definition in the same file, but it may cause over-sized excutable file. E.g.
class Foo
{
public:
template <typename T> void some_method(T t) {//...}
}
Also, it is possible to put template definition in the separate files, i.e. to put them in .cpp and .h files. All you need to do is to explicitly include the template instantiation to the .cpp files. E.g.
// .h file
class Foo
{
public:
template <typename T> void some_method(T t);
}
// .cpp file
//...
template <typename T> void Foo::some_method(T t)
{//...}
//...
template void Foo::some_method<int>(int);
template void Foo::some_method<double>(double);
Related videos on Youtube
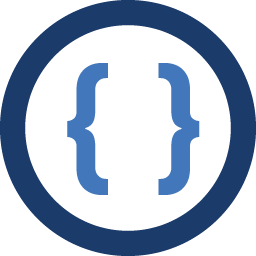
Admin
Updated on July 31, 2020Comments
-
Admin almost 4 years
I know it's possible to make a template function:
template<typename T> void DoSomeThing(T x){}
and it's possible to make a template class:
template<typename T> class Object { public: int x; };
but is it possible to make a class not within a template, and then make a function in that class a template? Ie:
//I have no idea if this is right, this is just how I think it would look class Object { public: template<class T> void DoX(){} };
or something to the extent, where the class is not part of a template, but the function is?
-
Benoît almost 15 yearsNot exactly true. The definition can be in a cpp file, as long as it is called once for each unique template parameter n-uplet from a non-template function/method after it has been defined.
-
Not Sure almost 15 yearsHence my "should" - keeping it in the header is the simplest way to accomplish that.
-
Patrick Johnmeyer almost 15 yearsActually, I believe you can explicitly specialize them, but you cannot partially specialize them. Unfortunately I don't know if this is a compiler-specific extension, or C++ standard.
-
Johannes Schaub - litb almost 15 yearsIt's actually standard c++. You can do struct A { template<typename> void f(); }; template<> void A::f<int>() { } for example. You just can't specialize them in class scope, but you can do so well when done in namespace scope. (not to be confused with the scope that the specialization is actually put into: the specialization will still be a member of the class - but its definition is done in namespace scope. Often the scope where something is put into is the same as the scope something is defined at - but that sometimes isn't true, as in all cases of out-of-class definitions)
-
MartianMartian almost 5 yearsgood example. but why template<typename T> is inside class definitino...???
-
CBK about 4 years@Martian2049 I believe this is so the template only applies to the member function within the class, and not the class as a whole. Exactly as the OP asked.