How to create a walking animation in LOVE 2D
You must understand how LÖVE works. It (very basically) does this:
love.load() -- invoke love.load just once, at the beginning
while true do -- loop that repeats the following "forever" (until game ends)
love.update(dt) -- call love.update()
love.draw() -- call love.draw()
end
This schema is so frequent that the loop itself has a name - it's called The Game Loop.
Your code does't work because you are using love.load()
as if it was part of the game loop, but it isn't. It's called at the beginning, during the first millisecond or so of your program, and never again.
You want to use love.load
do load the images, and love.update
to change them:
function love.load()
heroLeft = love.graphics.newImage("/hero/11.png")
heroRight = love.graphics.newImage("/hero/5.png")
heroDown = love.graphics.newImage("/hero/fstand.png")
heroUp = love.graphics.newImage("/hero/1.png")
hero = heroLeft -- the player starts looking to the left
end
function love.update(dt)
if love.keyboard.isDown("a") then
hero = heroLeft
elseif love.keyboard.isDown("d") then
hero = heroRight
elseif love.keyboard.isDown("s") then
hero = heroDown
elseif love.keyboard.isDown("w") then
hero = heroUp
end
end
function love.draw()
love.graphics.draw(background)
love.graphics.draw(hero, x, y)
end
The code above has certain repetitiveness that can be factored out using tables, but I've left it simple on purpose.
You will also notice that I have included the dt
parameter in the love.update
function. This is important, since you will need it to make sure that animations work the same in all computers (the speed at which love.update
is called depends on each computer, and dt
allows you to cope with that)
Nevertheless, if you want to do animations, you will probably want to use this Animation Lib or my own.
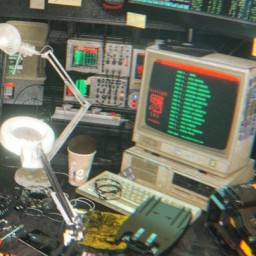
Switchkick
Updated on June 14, 2022Comments
-
Switchkick 12 months
So I was wondering how to change an image of character I've created depending on the key I've pressed/am pressing?
My ultimate going to to have a walking animation occuring when "d" (or any of the wasd keys) is pressed but then he stands still when the "d" key has just been pressed etc. All images have been created already.
I've tried this but it didn't work out:
function love.load() if love.keyboard.isDown("a") then hero = love.graphics.newImage("/hero/11.png") elseif love.keyboard.isDown("d") then hero = love.graphics.newImage("/hero/5.png") elseif love.keyboard.isDown("s") then hero = love.graphics.newImage("/hero/fstand.png") elseif love.keyboard.isDown("w") then hero = love.graphics.newImage("/hero/1.png") end function love.draw() love.graphics.draw(background) love.graphics.draw(hero, x, y) end