How to create an image on the fly with Node.js?
Solution 1
Maybe you could use canvas? There is also an implementation in node.js by Learnboost(TJ). I think this screencast is interesting to look at. As you see from presentation it even renders text in some examples. Also in the npm registry / node modules section I found a lot more interesting links
Solution 2
Here's some simple code using the canvas library:
const
fs = require("fs"),
{ createCanvas } = require("canvas");
const WIDTH = 100;
const HEIGHT = 50;
const canvas = createCanvas(WIDTH, HEIGHT);
const ctx = canvas.getContext("2d");
ctx.fillStyle = "#222222";
ctx.fillRect(0, 0, WIDTH, HEIGHT);
ctx.fillStyle = "#f2f2f2";
ctx.font = "32px Arial";
ctx.fillText("Hello", 13, 35);
const buffer = canvas.toBuffer("image/png");
fs.writeFileSync("test.png", buffer);
This is the resulting test.png
file:
To run it, you must first install the library:
npm i canvas
Instead of saving it to a file, you could, of course, send it as the response of an API call.
For more details on how to draw text using the canvas, see this MDN article.
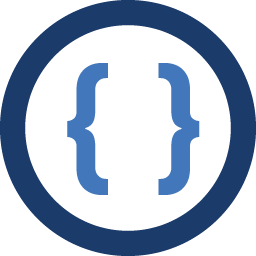
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I'm using Node.js and Express web framework, I need to create an on-the-fly image .png or .jpg (like captcha), then I have to send that image to the browser.
What is the simplest way to do that? In the image I should write letters/numbers (max 5).
P.S. I don't know what library to use that connects to an online service as the recaptcha module does.
-
Niso about 3 yearsHow would I get the results?
-
Lucio Paiva about 3 years@Niso if you mean the image, check the line
fs.writeFileSync("test.png", buffer);
, which writes the results to a file namedtest.png
. -
Niso about 3 yearsCool, thank you @Lucio. I did not notice that earlier.