How to create and use a JTextPane
Solution 1
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTextPane;
import javax.swing.text.BadLocationException;
import javax.swing.text.Style;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
public class Example {
public Example() {
JFrame frame = new JFrame();
JTextPane pane = new JTextPane();;
JButton button = new JButton("Button");
addColoredText(pane, "Red Text\n", Color.RED);
addColoredText(pane, "Blue Text\n", Color.BLUE);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pane.setPreferredSize(new Dimension(200, 200));
frame.getContentPane().add(pane, BorderLayout.CENTER);
frame.getContentPane().add(button, BorderLayout.WEST);
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
new Example();
}
public void addColoredText(JTextPane pane, String text, Color color) {
StyledDocument doc = pane.getStyledDocument();
Style style = pane.addStyle("Color Style", null);
StyleConstants.setForeground(style, color);
try {
doc.insertString(doc.getLength(), text, style);
}
catch (BadLocationException e) {
e.printStackTrace();
}
}
}
Try this example
Solution 2
This is a better start:
import javax.swing.*;
import javax.swing.text.*;
import java.awt.*;
public class test {
public test() throws BadLocationException {
JFrame frame = new JFrame();
DefaultStyledDocument document = new DefaultStyledDocument();
JTextPane pane = new JTextPane(document);
JPanel mainPanel = new JPanel();
JButton button = new JButton("Button");
button.setPreferredSize(new Dimension(100, 40));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pane.setPreferredSize(new Dimension(200, 200));
mainPanel.add(button);
frame.getContentPane().add(pane, BorderLayout.CENTER);
frame.getContentPane().add(mainPanel, BorderLayout.WEST);
StyleContext context = new StyleContext();
// build a style
Style style = context.addStyle("test", null);
// set some style properties
StyleConstants.setForeground(style, Color.BLACK);
document.insertString(0, "Four: success \n", style);
StyleConstants.setForeground(style, Color.RED);
document.insertString(0, "Three: error \n", style);
document.insertString(0, "Two: error \n", style);
StyleConstants.setForeground(style, Color.BLACK);
// add some data to the document
document.insertString(0, "One: success \n", style);
// StyleConstants.setForeground(style, Color.blue);
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) throws BadLocationException {
new test();
}
}
It is quite basic, but should get you started. You can create different methods when you have to add text and cascade through the colours.
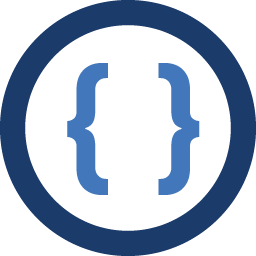
Admin
Updated on July 14, 2022Comments
-
Admin almost 2 years
I would like to create a program in Java that it is done this way:
A window containing a button and a component (JTextArea, JTextPane, etc.) that can't be modified in which appear some strings based on the execution of some work. As seen from the drawing, if the job is successful the text will be black, if there were errors will be marked red.
I managed to do everything correctly using a JTextArea and a JButton but I found that you can't change the color of the strings line by line.
I read that should use a JTextPane but I haven't been able to use it. Here's the code I wrote:
public class Example { public Example() { JFrame frame = new JFrame(); JTextPane pane = new JTextPane(); JButton button = new JButton("Button"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); pane.setPreferredSize(new Dimension(200, 200)); frame.add(pane); frame.add(button); frame.pack(); frame.setVisible(true); } public static void main(String[] args) { new Example(); } }
When I run the program, this is what is created:
Where the TextPane?
Also, before I added the text in JTextArea using append(), I haven't found a similar method to the JTextPane there? How do you use it? How do I change the color of a single line?
I have read and seen and tried various examples found on the internet but I could not finish anything... There are worked examples similar to this?
I apologize for the "generic" request...
Thanks
-
Computronium almost 4 yearsWhy do you add a null color style to the pane? Can that be done just once? And what does setting the color in StyleConstants do?