How to create my own strcpy function?
Solution 1
If you've already written a MyStrcpy, you're almost done:
The stpncpy() and strncpy() functions copy at most n characters from s2 into s1. If s2 is less than n characters long, the remainder of s1 is filled with `\0' characters. Otherwise, s1 is not terminated.
So you have a copy of strcpy that will stop after it has copied n characters; if it stopped earlier than that (b/c you got to the end of s2), fill the rest of s1 w/ /0's.
Solution 2
/* Include header files
*/
#include <stdio.h>
/* Pre=processor Directives
*/
#define word_size 20
/* Function prototype
*/
void my_func_strcpy(char *source, char* destination);
int main()
{
char source[word_size] = "I am source";
char destination[word_size] = "I am destination";
printf("The source is '%s' \n", source);
printf("The destination is '%s' \n", destination);
/* Calling our own made strcpy function
*/
my_func_strcpy(source, destination);
printf("The source data now is '%s' \n");
printf("The destination data now is '%s' \n");
}
/* Function to copy data from destination to source
*/
void my_func_strcpy(char *source, char* destination)
{
char temp[word_size] = {'\0'};
int index = 0;
/* Copying the destination data to source data
*/
while (destination[index] != '\0')
{
source[index] = destination[index];
index++;
}
/* Making the rest of the characters null ('\0')
*/
for (index = 0; index < word_size; index++)
{
source[index] = '\0';
}
}
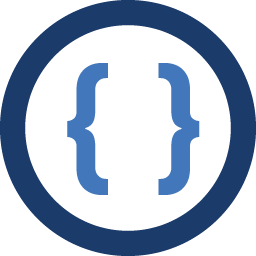
Admin
Updated on June 07, 2022Comments
-
Admin about 2 years
I am trying to design a program in which I will create a 3 functions that resemble functions in the c-standard library (strlen,strcmp,strcpy). The first two I have gotten close to finishing, only the last one is the main problem. I am trying to create a function that will have the same functionality as the standard function strcpy. Here is what I have so far.
void myStrncpy(char destination[], const char source[], int count) { for (int i = 0 ; source[i] != '\0' ; i++) { count++; } }
So far I have gotten the length of "source" and stored it in "count". What is the next step I need to take? I would prefer to use another for loop and if statements if possible. Thanks!
****EDIT****
This is what I have now...
void myStrncpy(char destination[], const char source[], int count) { for (int i = 0 ; source[i] != '\0' && destination[i] != '\0' ; i++) { destination[i] = source[i]; count++; }
}
OUTPUT:
str1 before: stringone str2 before: stringtwo str1 after : stringtwo str2 after : string two
2ND RUN (WHERE I'M GETTING MY PROBLEM):
str1 before: stringthree str2 before: stringfour str1 after: stringfoure str2 after: stringfour
What else do I need to put in my code so that it copies every letter until it runs out of room, or it copies every letter until it runs out of letters to copy?
-
Supernormal almost 8 yearsThat will copy from destination to source, won't it?
-
Azeemali Hashmani over 7 years@Supernormal yes it will. Also bro you are not emptying your last character When u copied str2 "stringfour" having length 10(not including '\0') into str1 which had "stringthree" having length 11 the last letter 'e' of str1 was not replaced and left out str1 after: stringfour 'e' (this was left out position 11, of previous string "stringthre'e') str2 after: stringfour
-
Tom Aranda over 6 yearsConsider explaining how this code answers the question.