How to create unselected indicator for tab bar in Flutter
7,455
Solution 1
You can use Stack/Container and TabBar together to make the underline
Stack(
fit: StackFit.passthrough,
alignment: Alignment.bottomCenter,
children: <Widget>[
Container(
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(color: colorSecondary, width: 2.0),
),
),
),
TabBar(
isScrollable: true,
onTap: (index) => tabsModel.value = listTabsModel[index],
tabs: listTabsModel
.map(
(value) => Tab(
child: value.tabComponent,
),
)
.toList(),
),
],
);
TabBar with an underline for unselected
Not exactly what you are looking for but it can give the underline for un-selected tab.
Solution 2
Instead of using container in tabs, try this (wrapping the TabBar with DecoratedBox and providing bottom border
DecoratedBox(
//This is responsible for the background of the tabbar, does the magic
decoration: BoxDecoration(
//This is for background color
color: Colors.white.withOpacity(0.0),
//This is for bottom border that is needed
border: Border(bottom: BorderSide(color: Colors.grey, width: 0.8)),
),
child: TabBar(
controller: _controller,
tabs: [
...
],
),
)
Will not look exactly as you want, but will give underlined indication to unselected tabs.
Solution 3
Solution that I used is similar to what @andy suggested, but a bit different
Stack(
children: [
Container(
// The height of TabBar without icons is 46 (72 with), so 2 pixels for border
height: 48,
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(
color: Colors.red,
width: 2,
),
),
),
),
TabBar(
isScrollable: true,
indicator: UnderlineTabIndicator(
borderSide: BorderSide(
color: Colors.yellow,
width: 2.0,
),
),
indicatorSize: TabBarIndicatorSize.tab,
tabs: <Widget>[
Tab(
text: "Tab 1",
),
Tab(
text: "Tab 2",
),
Tab(
text: "Tab 3",
)
],
),
],
)
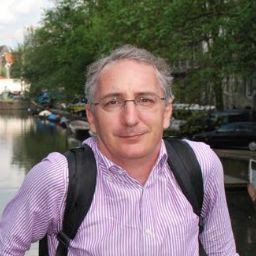
Author by
Alexandre Fett
Updated on December 06, 2022Comments
-
Alexandre Fett over 1 year
I created a custom indicator for tab bar using Decorator. I want to create a unselected indicator for not selected tabs in tab bar.
I did a container with custom decoration but current selected indicator draws behind container decoration.
new TabBar( labelColor: Colors.black, unselectedLabelColor: Colors.grey, indicator: new CustomTabIndicator(), tabs: [ new Container(decoration: new CustomTabInactive(),child: Tab(icon: Icon(Icons.person )))])
-
ulusoyca over 3 yearsWe shouldn't set a fixed height. We can't be sure of the AppBar height. Any constant in the source code is subject to change and might differ from platform to platform.