How to declare an array of an object statically
Solution 1
Assuming you have public fields/properties on your class:
class MyObject
{
public int i1 { get; set; }
public string s1 { get; set; }
public double d1 { get; set; }
} // note: no semicolon needed here
static MyObject[] myO = { new MyObject { i1 = 1, s1 = "1", d1 = 1.0 },
new MyObject { i1 = 2, s1 = "2", d1 = 2.0 },
};
Solution 2
You need to fill the array with object instances.
Create a constructor that takes parameters, then write
new MyObject[] { new MyObject(a, b, c), new MyObject(d, e, f) }
Solution 3
You'll have to initialize the array with new object instances.
class MyObject
{
int i1;
string s1;
double d1;
public MyObject(int i, string s, double d)
{
i1 = i;
s1 = s;
d1 = d;
}
};
static MyObject[] myO = new MyObject[] {
new MyObject(1, "1", 1.0),
new MyObject(2, "2", 2.0)
};
Unfortunately there is no way to specify custom initializers like they are for arrays of built-in types or dictionaries. For (future) reference of what I mean:
int[] arr = { 1, 2, 3, 4 };
var list = new List<string> { "abc", "def" };
var dict = new Dictionary<string, int> { { "abc", 1 }, { "def", 2 } };
Solution 4
You'll need to instantiate the objects in the array:
static MyObject[] myO = new MyObject
{
new MyObject { i1 = 1, s1 = "1", d1 = 1.0 },
new MyObject { i1 = 2, s1 = "2", d1 = 2.0 },
};
Solution 5
It is exactly what you want but you can achieve your goal with following code:
class MyObject
{
public int i1;
public string s1;
public double d1;
};
static MyObject[] myO = new[] { new MyObject { i1=1, s1="1", d1=1.0 }, new MyObject { i1=2, s1="2", d1=2.0 } };
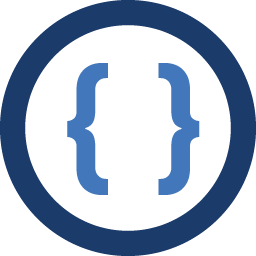
Admin
Updated on May 25, 2020Comments
-
Admin almost 4 years
I'm a long time C programmer but new to C#. I want to declare an object then creating an array of that object filling it statically (I have a very large table to enter). For example
class MyObject { int i1; string s1; double d1; }; static MyObject[] myO = new MyObject {{1,"1",1.0}, {2,"2",2.0}};
This doesn't work but you get the idea. Any help appreciated.