How to decode a cookie secret, set using Connect's cookieSession?
Hmmm... you seem to be a bit confused about the use of cookie sessions.
Your
/setcookie
path doesn't set any cookie/session values. To use a cookie session, you need to set some values onreq.session
, for examplereq.session.message = "Hi there!"
These values are now stored in the cookie.You can't
res.app.use()
in just one callback, the request session won't work anywhere else this way. To read those cookies in another request, put theapp.use(express.cookieSession({ key: "test", secret: "test" }));
at the application level.
Edit: Actually I've thought about your res.app.use() call and it could break your app / cause memory to leak by adding more and more middleware layers (cookieSession) to your app, each time someone requests /setcookie
. If you want to only add the cookieSession middleware in specific requests, you need to do the following:
yourParser = express.cookieSession({ key: "test", secret: "test" });
app.get('/', yourParser, ...);
app.get('/setcookie', yourParser, ...);
Solution
This is the actual fixed source:
app.use(express.bodyParser());
app.use(express.cookieParser());
app.use(express.cookieSession({ key: "test", secret: "test" }));
app.get('/setcookie', function(req, res, next){
req.session.message = "Hellau there";
res.end();
});
Now to inspect those values, in app.get('/',...)
try this:
app.get('/', function(req, res, next){
console.log(req.session); //Yay, this will be available in all requests!
res.end();
});
Debugging
And to answer the question of how to manually decode what's stored in a cookie, look in connect/lib/middleware/cookieSession.js
:
// cookieParser secret
if (!options.secret && req.secret) {
req.session = req.signedCookies[key] || {};
} else {
// TODO: refactor
var rawCookie = req.cookies[key];
if (rawCookie) {
var unsigned = utils.parseSignedCookie(rawCookie, secret);
if (unsigned) {
var originalHash = crc16(unsigned);
req.session = utils.parseJSONCookie(unsigned) || {};
}
}
}
It takes req.cookies[key]
(basically what you've been doing already (req.cookies.test
)), pops it into utils.parseSignedCookie
and pops that into utils.parseJSONCookie
.
I put your raw cookie string into the utils.js file at the end:
var signed = exports.parseSignedCookie(
's:j:{}.8gxCu7lVJHVs1gYRPFDAodK3+qPihh9G3BcXIIoQBhM'
, 'test');
var parsed = exports.parseJSONCookie(signed);
console.log(parsed);
and ran that. Guess what I got:
{}
Why? Because you never set anything on the req.session
object. :)
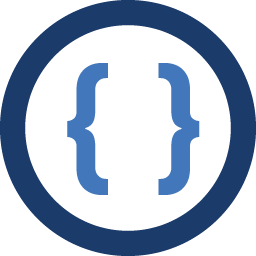
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am setting a cookie using Connect's
cookieSession()
. This seems to encrypt the cookie's secret value, returning something like 's:j:{}.8gxCu7lVJHVs1gYRPFDAodK3+qPihh9G3BcXIIoQBhM'How do I decrypt the cookie's value?
Example code:
app.use(express.bodyParser()); app.use(express.cookieParser()); app.get('/setcookie', function(req, res, next){ res.app.use(express.cookieSession({ key: "test", secret: "test" })); console.log(req.cookies.test); res.end(); }); app.get('/', function(req, res, next){ console.log(req.cookies.test); res.end(); });