How to decrypt of the encrypted value in dart?
Solution 1
An HMAC is a message authentication code. It is a digest used to verify that a message has not been tampered with, similar to a signature. It is constructed using a one-way hash function, in this case SHA256. It is not an encryption, and it cannot be reversed, therefore the value you have cannot be decrypted.
The only thing you can do with an HMAC is, given the HMAC and the corresponding plain-text, verifying the origin of the plain-text and that it has not been tampered with.
Looking at the GitHub page for the Dart crypto library, it looks like it only supports digest algorithms. There are no encryption algorithms listed, so you will need to use a different library if you want to do two-way encryption which can actually be decrypted. The Cipher library looks like it could be promising in this regard.
Solution 2
The easiest way IMO is to use encrypt:
import 'package:encrypt/encrypt.dart';
final key = Key.fromUtf8('put32charactershereeeeeeeeeeeee!'); //32 chars
final iv = IV.fromUtf8('put16characters!'); //16 chars
//encrypt
String encryptMyData(String text) {
final e = Encrypter(AES(key, mode: AESMode.cbc));
final encrypted_data = e.encrypt(text, iv: iv);
return encrypted_data.base64;
}
//dycrypt
String decryptMyData(String text) {
final e = Encrypter(AES(key, mode: AESMode.cbc));
final decrypted_data = e.decrypt(Encrypted.fromBase64(text), iv: iv);
return decrypted_data;
}
Solution 3
PointyCastle (https://pub.dartlang.org/packages/pointycastle) has been recommended in the past, but it appears to have not yet been updated for Dart 2. :(
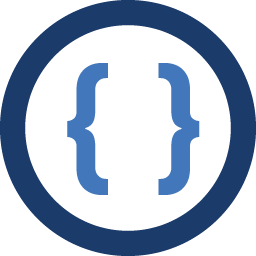
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
I am testing the Cryptographic hashing functions for Dart. I can't find any information about DECRYPTION? Can anyone show me how to decryption of the encrypted value?
And this is example;
import 'dart:convert'; import 'package:crypto/crypto.dart'; void main() async { var key = utf8.encode('p@ssw0rd'); var bytes = utf8.encode("Dart and Aqueduct makes my life easier. Thank you."); // TODO: ENCRYPTION var hmacSha256 = new Hmac(sha256, key); // HMAC-SHA256 var digest = hmacSha256.convert(bytes); print(“————ENCRYPTION—————“); print("HMAC digest as bytes: ${digest.bytes}"); print("HMAC digest as hex string: $digest"); print('\r\n'); // TODO: DECRYPTION ???????????? print(“————DECRYPTION—————“); print(?????????); }
-
Admin almost 6 yearsThanks Sean, The Cipher is very old and didn't pass the documentation. So, do you know anything newer than Chiper, special from dart itself?
-
Sean Burton almost 6 yearsSorry, I don't really use Dart myself so I'm not familiar with the libraries that are available, and I can't seem to find any other options on Google.
-
Admin almost 6 yearsThanks Sean, I will check later
-
Admin almost 6 yearsWell, thanks to Dart community. Leo Cavalcante did the nice job. Its new and improving. Currently this one is perfect to make 2 way secure communication between Flutter Mobile App and Aqueduct web api. pub.dartlang.org/packages/encrypt
-
Sean Burton over 3 yearsIf your application is to be used in anything that requires real security, please don't roll your own encryption algorithm, especially not one as incredibly flawed as this.