How to define an explicit constructor for an url object in Java
Solution 1
You just need to define a default constructor for your class which throws MalformedURLException:
class MyClass {
private URL url = new URL("http://www.google.com");
public MyClass() throws MalformedURLException {}
}
The error is happening because the constructor Java is generating for your class isn't able to throw anything, you must specify that it might.
Edit
Seeing your code, your other option is this (I assume setup() gets called before you use the URL)
URL url;
void setup() {
try {
url = new URL("http://www.google.com");
} catch (MalformedURLException ex) {
throw new RuntimeException(ex);
}
}
Solution 2
Since the URL
constructor throws a checked exception you have to either catch it or rethrow it. You can create your own factory method that just wraps it in a RuntimeException
:
public class URLFactory {
public static URL create(String urlString) {
try {
return new URL(urlString);
} catch (MalformedURLException e) {
throw new RuntimeException(e);
}
}
}
Or maybe just this in your environment?
URL createURL(String urlString) {
try {
return new URL(urlString);
} catch (MalformedURLException e) {
throw new RuntimeException(e);
}
}
And use it instead:
URL base = URLFactory.create("http://www.google.com");
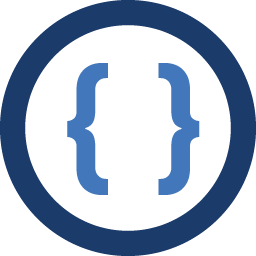
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I am defining a url in processing (or java) with the line:
URL base = new URL("http://www.google.com/");
So I get the following error: Default constructor cannot handle exception type MalformedURLException thrown by implicit super constructor. Must define an explicit constructor. Which I assume is because if my url isn't valid, there's no corresponding
catch
to catch the error (because I'm declaring my url outside oftry
).But, i want to define this url outside of
try
, because my maintry
block is in a loop and the url is static. So there's no need to do the definition of the url more than once (and because it's static i'm not afraid of any MalformedURLExceptions).How do I do that? Is there really no other way than to define the url within a separate
try
block? (because that seems a bit too much for just a simple static url)
// import libraries import java.net.*; import java.io.*; // url URL base = new URL("http://www.google.com"); void setup() { } void draw() { try { // retrieve url String[] results = loadStrings(base); // print results println(results[0]); } catch (MalformedURLException e) { e.printStackTrace(); } catch (ConnectException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } // stop looping noLoop(); }