How to delete a file from a SFTP server programmatically using SharpSSH?
12,738
Solution 1
The SshExec class didn't work for me, but a little Reflection magic worked:
var prop = sftp.GetType().GetProperty("SftpChannel", BindingFlags.NonPublic | BindingFlags.Instance);
var methodInfo = prop.GetGetMethod(true);
var sftpChannel = methodInfo.Invoke(sftp, null);
((ChannelSftp) sftpChannel).rm(ftpPath);
Solution 2
To accomplish this you will need to modify the SharpSSH assembly to expose the functionality you require.
Obtain the source code and open $\SharpSSH-1.1.1.13.src\SharpSSH\Sftp.cs
Insert the following lines of code before the end of the class:
public void Delete(string path)
{
SftpChannel.rm(path);
}
Recompile and reference the recompiled DLL in your project. You will now be able to delete files on the SFTP server.
Solution 3
Well you can also use SshExec class and then execute the "rm" command using "RunCommand" method. This way you wont have to recompile and build a new dll.
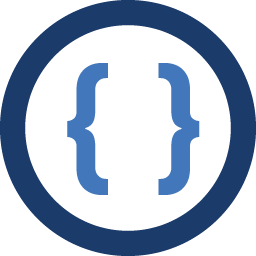
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
How to delete a file from a SFTP server using Tamir Gal's SharpSSH? I have been able to accomplish other functionality but deletion.