How to delete cookie from .Net
84,980
Solution 1
HttpCookie currentUserCookie = HttpContext.Current.Request.Cookies["currentUser"];
HttpContext.Current.Response.Cookies.Remove("currentUser");
currentUserCookie.Expires = DateTime.Now.AddDays(-10);
currentUserCookie.Value = null;
HttpContext.Current.Response.SetCookie(currentUserCookie);
It works.
Solution 2
Instead of adding the cookie, you should change the Response's
cookies Expires
to a value in the past:
if (Request.Cookies["currentUser"] != null)
{
Response.Cookies["currentUser"].Expires = DateTime.Now.AddDays(-1);
}
Sidenote: Instead of throw ex
you should just throw
it to keep its stacktrace. C#: Throwing Custom Exception Best Practices
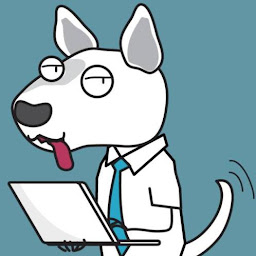
Author by
Merrizee
Updated on July 20, 2022Comments
-
Merrizee almost 2 years
Possible Duplicate:
Delete cookie on clicking sign outI want to delete cookies when the user logout.
Here is my code:
if (HttpContext.Current.Request.Cookies["currentUser"] != null) { DeleteCookie(HttpContext.Current.Request.Cookies["currentUser"]); } public void DeleteCookie(HttpCookie httpCookie) { try { httpCookie.Value = null; httpCookie.Expires = DateTime.Now.AddMinutes(-20); HttpContext.Current.Request.Cookies.Add(httpCookie); } catch (Exception ex) { throw (ex); } }
But it doesn't work. Do you have any suggestion?
-
Dykam over 11 yearsWhy catch the exception anyway if nothing is done with it?
-
Merrizee over 11 yearsI tried but it doesn't work. Still I can see the user name after logout. httpCookie.Expires = DateTime.Now.AddDays(-1);
-
S.. over 11 yearsSorry...i did a quick search and found this msdn.microsoft.com/en-us/library/ms178195.aspx May be this might be useful to u.
-
Merrizee over 11 yearsAs you can see in my question, I already set the cookie's expiration time to past. But the same result.
-
Tim Schmelter over 11 years@Dykam: You can decide later to log it or do whatever you want. I often add a
TODO: implement logging
there in the first version. On this way you have at least added the try/catch to remember that this might cause an exception. -
serge over 8 yearsWhat if I want to delete a cookie, then create an other cookie with the same name? Will the Expires act on my new created cookie?
-
James Wilkins almost 7 yearsI know this is an old answer, but for newcomers, I don't think removing the cookie first does anything useful, since it is set again later anyhow.
-
Luca Ritossa about 5 yearsI can confirm what @JamesWilkins said. The
Remove
is not needed since the cookie is overwritten. -
Janspeed almost 5 yearsAccording to this docs.microsoft.com/en-us/dotnet/api/… you should use HttpContext.Current.Response.Set instead of HttpContext.Current.Response.SetCookie not sure about the difference though.
-
MikeDev over 3 yearsI found that I needed to have the Remove line - otherwise I had a lot of expired cookies of the same name lingering around, and when it came time to read the value, one of the old "expired" incarnations was being read