How to delete section from UITableView (iPhone/iPad)
Solution 1
Implementing your own headerView is the way to go. Apple does not provide a built-in user interface to delete sections. If you pull down the notification area of an iOS device you get an idea how to make it look good.
The implementation is not very complicated either. I used something like this:
- (CGFloat)tableView:(UITableView *)tableView heightForHeaderInSection:(NSInteger)section {
return 21.0f;
}
- (UIView *)tableView:(UITableView *)tableView viewForHeaderInSection:(NSInteger)section {
UIView *headerView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 320, 21)];
headerView.backgroundColor = [UIColor lightGrayColor];
UILabel *headerLabel = [[UILabel alloc] initWithFrame:CGRectMake(8, 0, 250, 21)];
headerLabel.text = [NSString stringWithFormat:@"Section %d", section];
headerLabel.font = [UIFont boldSystemFontOfSize:[UIFont systemFontSize]];
headerLabel.textColor = [UIColor whiteColor];
headerLabel.backgroundColor = [UIColor lightGrayColor];
[headerView addSubview:headerLabel];
UIButton *button = [UIButton buttonWithType:UIButtonTypeCustom];
button.tag = section + 1000;
button.frame = CGRectMake(300, 2, 17, 17);
[button setImage:[UIImage imageNamed:@"31-circle-x"] forState:UIControlStateNormal];
[button addTarget:self action:@selector(deleteButtonPressed:) forControlEvents:UIControlEventTouchUpInside];
[headerView addSubview:button];
return headerView;
}
- (IBAction)deleteButtonPressed:(UIButton *)sender {
NSInteger section = sender.tag - 1000;
[self.objects removeObjectAtIndex:section];
[self.tableView deleteSections:[NSIndexSet indexSetWithIndex:section] withRowAnimation:UITableViewRowAnimationAutomatic];
// reload sections to get the new titles and tags
NSInteger sectionCount = [self.objects count];
NSIndexSet *indexes = [NSMutableIndexSet indexSetWithIndexesInRange:NSMakeRange(0, sectionCount)];
[self.tableView reloadSections:indexes withRowAnimation:UITableViewRowAnimationNone];
}
Solution 2
Yes there are existing methods available to delete section in tableview.You can use following methods:
[m_TableView beginUpdates];
[m_TableView deleteSections:[NSIndexSet indexSetWithIndex:1] withRowAnimation:UITableViewRowAnimationTop];
[m_TableView endUpdates];
But you have to keep one thing in mind when you delete section you have to modify:
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
because now your section number is reduced by 1.
Solution 3
If I am correct, Apple only provide row delete in the UITableView edit mode.
I can think of 3 ways of adding a button to delete a specific section.
First, put a button besides a section of the UITableView
, and it will be not inside the UITableView
(I don't think you can put a button besides the section title inside the UITableView
, or maybe you could but I don't know how to do it). You can check whether the UITableView
is in editable mode and show or hide your button accordingly.
Seond, If you do want a delete button in the UITableView
, you can create cuntom UITableViewCell and put the section delete button inside the cell. That way you will have a button in every UITableViewCell
, which is not that great.
Third choice would be not providing any button, just let user delete the cell in the Apple default way. What you do is just keep tracking how many objects left in your array used to populate the section. If the count is get to 0, then remove the section.
Here is what I did before to do the similar thing:
I have an NSMutableArray
firstLevelArray contains a couple of NSMutableArray
secondLevelArray inside with each contains some objects. So every secondLevelArray will provide data for a section and an object in secondLevelArray will provide data for every cell. In - (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
, I do return [firstLevelArray count];
and wherever I wanna delete a section, after [firstLevelArray removeObjectAtIndex:someIndex]
, I do [myTableView reloadData]
Hope this helps
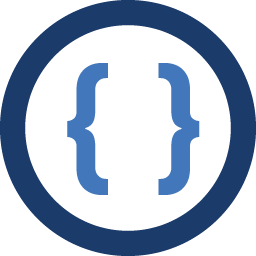
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I'm trying to come up with a nice (in terms of coding & apperance) way of deleting a section from a table view. Ideally I would like to create a way of deleting sections that is similar to that of the process for deleting a
UITableViewCell
(ie. the red minus button that shows the red delete button when pressed).Is there any pre-existing solution to this problem? I have tried doing a bit of research into the are but I can't really find anything of much use. If there isn't a pre-existing solution I was thinking I could try and implement one. My plan would be to create a custom header for each section. Within the custom header I'd add the delete buttons that would react the same way as when deleting a row. Do people think this would work?
So to be clear, I'm asking whether there is an existing solution for deleting sections in a
UITableView
. If there isn't then I'd like to know if people think my idea would be feasible or if there are any issues I've overlooked with it.Thanks in advance.