how to detect a proxy using javascript
Solution 1
You could make an ajax request to a known external server (google.com) and then get the headers out of that request to see if the proxy headers are in the request...
var proxyHeader = 'via';
var req = new XMLHttpRequest();
req.open('GET', document.location, false);
req.send();
var header = req.getResponseHeader(proxyHeader);
if (header) {
// we are on a proxy
}
Change proxyHeader
to what ever your proxy adds to the response.
EDIT: You will have to add a conditional for supporting the IE implementation of XMLHttpRequest
EDIT:
I am on a proxy at work and I have just tested this code in jsfiddle and it works. Could be made prettier so that is supports IE and does an async get but the general functionality is there... http://jsfiddle.net/unvHW/
It turns out that detecting 'via' is much better...
Solution 2
Note that this solution will not work on every proxy and would probably only work if you are BEHIND the proxy :
Some proxies append a field in the response headers of an HTTP request which is called : X-Forwarded-For
Maybe you can achieve what you are trying to do with an AJAX request to google.com
for example and check if the field is there.
Something like this :
$.ajax({
type: 'POST',
url:'http://www.google.com',
data: formData,
success: function(data, textStatus, request){
if(request.getResponseHeader('X-Forwarded-For')) !== undefined)
alert("Proxy detected !");
}
});
Edit: As Michael said, the X-Forwarded-For is only appended to requests. You'd better check for the response header your proxy puts in the response header.
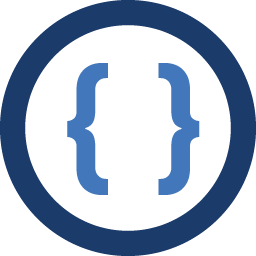
Admin
Updated on July 01, 2022Comments
-
Admin almost 2 years
in a web page, is there a way to detect by javascript if a web browser is using a PAC file http://xxx.xx.xx.xxx/toto.pac ? Notes : the same page can be viewd behind many PACs, i don't want to use a server end language, i can edit the toto PAC file if necessary. Regards