How to detect user has stopped typing in TextField?
Solution 1
Thanks to @pskink
I was able to achieve the functionality I was looking for.
import stream_transform package in your pubspec.yaml
stream_transform: ^0.0.19
import 'package:stream_transform/stream_transform.dart';
StreamController<String> streamController = StreamController();
@override
void initState() {
streamController.stream
.transform(debounce(Duration(milliseconds: 400)))
.listen((s) => _validateValues());
super.initState();
}
//function I am using to perform some logic
_validateValues() {
if (textController.text.length > 3) {
// code here
}else{
// some other code here
}
}
TextField code
TextField(
controller: textController,
onChanged: (val) {
streamController.add(val);
},
)
Solution 2
In my case I also required flutter async.
//pubspec.yaml
stream_transform: ^2.0.0
import 'dart:async';
import 'package:stream_transform/stream_transform.dart';
StreamController<String> streamController = StreamController();
// In init function
streamController.stream
.debounce(Duration(seconds: 1))
.listen((s) => {
// your code
});
// In build function
TextField(
style: TextStyle(fontSize: 16),
controller: messageController,
onChanged: (val) {
myMetaRef.child("isTyping").set(true);
streamController.add(val);
},
)
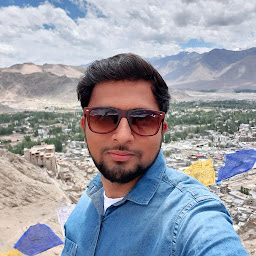
Vicky Salunkhe
Full Stack Developer Interested in solving complex problems and building real-world solutions. 👨🏻💻🏆🙌🏻 Visit vickysalunkhe.in to know more about me. 👨🏻💻 My LinkedIN Profile 👉🏻 Linked In
Updated on December 12, 2022Comments
-
Vicky Salunkhe over 1 year
I am using a
TextField
under whichonChanged
functionI am calling my code but the issue right now is the code gets execute everytime a new word is either entered or deleted.
what I am looking for is how to recognize when a user has stopped typing.
means adding some delay or something like that.
I have tried adding
delay
also usingFuture.delayed
function but that function also gets executed n number of times.TextField( controller: textController, onChanged: (val) { if (textController.text.length > 3) { Future.delayed(Duration(milliseconds: 450), () { //code here }); } setState(() {}); }, )