How to determine which version of Windows?
13,576
Solution 1
You're looking for the Environment.OSVersion
, Environment.Is64BitProcess
, and Environment.Is64BitOperatingSystem
properties.
Before .Net 4.0, you can check whether the process is 64-bit by checking whether IntPtr.Size
is 8
, and you can check whether the OS is 64-bit using this code:
public static bool Is64BitProcess
{
get { return IntPtr.Size == 8; }
}
public static bool Is64BitOperatingSystem
{
get
{
// Clearly if this is a 64-bit process we must be on a 64-bit OS.
if (Is64BitProcess)
return true;
// Ok, so we are a 32-bit process, but is the OS 64-bit?
// If we are running under Wow64 than the OS is 64-bit.
bool isWow64;
return ModuleContainsFunction("kernel32.dll", "IsWow64Process") && IsWow64Process(GetCurrentProcess(), out isWow64) && isWow64;
}
}
static bool ModuleContainsFunction(string moduleName, string methodName)
{
IntPtr hModule = GetModuleHandle(moduleName);
if (hModule != IntPtr.Zero)
return GetProcAddress(hModule, methodName) != IntPtr.Zero;
return false;
}
[DllImport("kernel32.dll", SetLastError=true)]
[return:MarshalAs(UnmanagedType.Bool)]
extern static bool IsWow64Process(IntPtr hProcess, [MarshalAs(UnmanagedType.Bool)] out bool isWow64);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError=true)]
extern static IntPtr GetCurrentProcess();
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
extern static IntPtr GetModuleHandle(string moduleName);
[DllImport("kernel32.dll", CharSet = CharSet.Ansi, SetLastError=true)]
extern static IntPtr GetProcAddress(IntPtr hModule, string methodName);
Solution 2
Take a look at Environment.OSVersion
and Environment.Is64BitOperatingSystem
Solution 3
You could do
System.OperatingSystem osInfo = System.Environment.OSVersion;
Have a look at this.
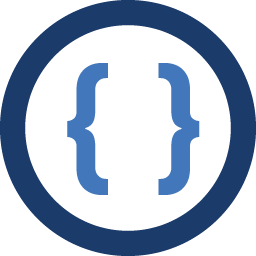
Author by
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
- How to determine which version of Windows? WinXP, Vista or 7 etc.
- 32 or 64 bit?
UPD: for .Net 2.0 - 3.5
-
Grant Thomas almost 13 yearsAFAIK, the
OperatingSystem
type directly doesn't expose a field to determine the bitiness. Some validation against known terms would need to take place, methinks. -
SLaks almost 13 years@MrD:
Is64BitOperatingSystem
-
Grant Thomas almost 13 years@Slaks: Ya, just noticed the update - had no idea
Environment
housed such a property. Nice one. This must be new to .NET4? -
Admin almost 13 yearsMy Windows Version is "Microsoft Windows NT 6.1.7600.0". How determine it XP, Vista or 7?
-
SLaks almost 13 yearsXP is 5.1, Vista is 6.0, and 7 is 6.1
-
Admin almost 13 yearsWhich version for Win2003 and Win2008?
-
SLaks almost 13 yearsThey're the same as XP and Vista.