How to disable Chrome autocomplete feature?
Solution 1
Do autocomplete="new-password"
to disable autocomplete.
Solution 2
You can override chrome autofill by add onFocus
attribute.
render()
{
return <input type="text" name="name" value="this is my input" autoComplete="off" onFocus={this.onFocus} />
}
In the onFocus
method we need to change "autocomplete" attribute through javaScript.
onFocus = event => {
if(event.target.autocomplete)
{
event.target.autocomplete = "whatever";
}
};
This solution works for me.
let me know if it works for you ;)
Solution 3
Nothing worked for me including new-password
, so this is how I did:
onFocus={(event) => {
event.target.setAttribute('autocomplete', 'off');
console.log(event.target.autocomplete);
}}
Solution 4
I got the same issue with my React project. My solution is to use a random string for autoComplete attribute. Don't use "off", as per Pim, you need to set a invalid value to really turn auto completion off. Please also note the attribute name has to be autoComplete in React.
Solution 5
When using jsx - you have to camel case attributes; so autoComplete="new-password"
instead of autocomplete
.
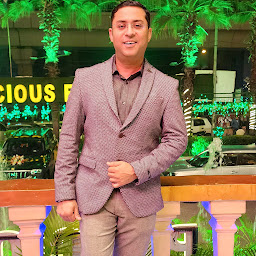
Shiva Wahi
Updated on July 05, 2022Comments
-
Shiva Wahi almost 2 years
We want to disable autocomplete in Chrome browser in our React JavaScript application. We have tried a bunch of solutions available on the Internet but nothing worked.
autoComplete=off
is not reliable and so are other ways.This is really important for us at this moment so can you please suggest us a foolproof way to disable autocomplete in Chrome using React JavaScript?
Secondly, we are using a common control/component for our text boxes and using them everywhere