How to disable div blocks having a certain id pattern?
Solution 1
If it has an id
, you can use document.getElementById
:
var div = document.getElementById("alertPanel");
Then if it exists, you can either remove it (probably a bad idea) or hide it:
if (div) {
div.style.display = "none"; // Hides it
// Or
// div.parentNode.removeChild(div); // Removes it entirely
}
Update: Re your comment on another answer:
thanks for your answer. Does your statemt apply to a page with iframes too. The div in question is in an iframe. I've tried ypur solution and it didn't work unfortunately. maybe a link to the page will help: tennis.betfair.com the div i want to disable is the one with id: minigamesContainer
If the element is in an iframe
, then you have to call getElementById
on the document that's in the iframe
, since iframe
s are separate windows and have separate documents. If you know the id
of the iframe
, you can use document.getElementById
to get the iframe
instance, and then use contentDocument
to access its document, and then use getElementById
on that to get the "minigamesContainer" element:
var iframe, div;
iframe = document.getElementById("the_iframe_id");
if (iframe) {
try {
div = iframe.contentDocument.getElementById("minigamesContainer");
if (div) {
div.style.display = "none";
}
}
catch (e) {
}
}
(The try/catch
is there because of a potential security error accessing the content of the iframe; I don't know Greasemonkey well enough to know whether the SOP applies to it. I tend to assume it doesn't, but better safe...)
If you don't know the id
of the iframe
or if it doesn't have one, you can just loop through all of them by getting them with document.getElementsByTagName
and then looping:
var iframes, index, iframe, div;
iframes = document.getElementsByTagName("iframe");
for (index = 0; index < iframes.length; ++index) {
iframe = iframes[index];
try {
div = iframe.contentDocument.getElementById("minigamesContainer");
if (div) {
div.style.display = "none";
break;
}
}
catch (e) {
}
}
References:
Solution 2
In valid HTML you can have only element with certain ID. So:
document.getElementById('alertPanel').style.visiblity = 'hidden';
But if you still need to iterate all div's and check their ID's, then this should work:
if (myDivs[i].id == "alertPanel") myDivs[i].style.visibility = 'hidden';
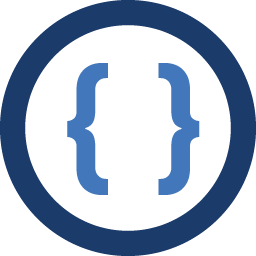
Admin
Updated on June 21, 2022Comments
-
Admin almost 2 years
I would like to write a greasemonkey script to disable a div on a certain page. On any given load of the page I don't know where in the DOM the div will be but I know it's always called
<div id = "alertPanel"> ....</div>
How would I go about disabling this div?
My intial thoughts were something along the lines of:
var myDivs= document.getElementsByTagName('div'); for (i=0; i<myDivs.length; i++) { if (myDivs[i].<get id property somehow> = "alertPanel") myDivs[i].style.visibility = 'hidden'; }
but as you can tell I'm stuck at accessing the id property for an equality check.
Incidently, I'm using a text editor to write this - I guessing that a standard javascript editor would give an autocompletion list after typing in
myDivs[i].