How to disable java mail trace on console when sending an email
Solution 1
In spring this worked for me:
<property name="javaMailProperties">
<props>
<prop key="mail.transport.protocol">smtp</prop>
<prop key="mail.smtp.auth">false</prop>
<prop key="mail.smtp.starttls.enable">true</prop>
<prop key="mail.debug">false</prop>
</props>
</property>
tested with versions 1.4.7+
Solution 2
It has nothing to do with setDebug
method!
I was using version 1.40 of javax.mail library and had the exact same problem. There is this System.out.println(">>>>>Sending data " + data + "<<<<<<");
line of code in the SMTPTransport
class which has nothing to do with debug logs and always writes its data in console!
Simply go get version 1.5 of the library and the problem will be solved.
You can get it from Maven repository.
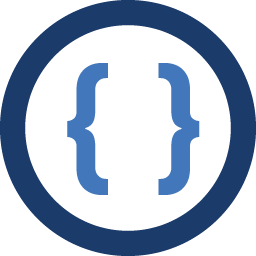
Admin
Updated on August 10, 2022Comments
-
Admin over 1 year
Here is my code for sending email:
public void sendMail() { try { // Propiedades de la conexión Properties props = new Properties(); props.put("mail.transport.protocol", "smtp"); props.put("mail.smtp.host", SMTP_HOST_NAME); props.put("mail.smtp.port", SMTP_PORT); props.put("mail.smtp.auth", "true"); Authenticator auth = new SMTPAuthenticator(); Session mailSession = Session.getDefaultInstance(props, auth); Transport transport = mailSession.getTransport(); MimeMessage message = new MimeMessage(mailSession); Multipart multipart = new MimeMultipart("alternative"); BodyPart text = new MimeBodyPart(); text.setContent(mailMessage,"text/html; charset=UTF-8"); multipart.addBodyPart(text); message.setContent(multipart); if(friendlyName != null){ //String send = friendlyName + " <" + sender + ">"; message.setFrom(new InternetAddress(sender, friendlyName)); }else{ message.setFrom(new InternetAddress(sender)); } message.setSubject(subject,"UTF-8"); message.addRecipient(Message.RecipientType.TO, new InternetAddress(receiver)); transport.connect(); transport.sendMessage(message, message.getRecipients(Message.RecipientType.TO)); transport.close(); }catch (Exception e) { // TODO: handle exception } }
When I send an email I get this output on console (or catalina.out):
>>>>>Sending data EHLO xxxxxx<<<<<<
>>>>>Sending data AUTH LOGIN<<<<<<
>>>>>Sending data xxxxxxxxxxxxxxxxxxxx<<<<<<
>>>>>Sending data xxxxxxxxxxxxxx<<<<<<
>>>>>Sending data MAIL FROM: <<<<<<
>>>>>Sending data RCPT TO: <<<<<<
>>>>>Sending data DATA<<<<<<
>>>>>Sending data <<<<<<
>>>>>Sending data .<<<<<<
>>>>>Sending data QUIT<<<<<<
How can I disable this output? My catalina.out is growing too fast. Setdebug(false) method does not solve my problem.