How to display a non-modal CDialog?
Solution 1
/* CChildDialog class is inherited from CDialog */
CChildDialog *m_pDialog = NULL;
// Invoking the Dialog
m_pDialog = new CChildDialog();
if (m_pDialog != NULL)
{
BOOL ret = m_pDialog->Create(IDD_CHILDDIALOG, this);
if (!ret) //Create failed.
{
AfxMessageBox(_T("Error creating Dialog"));
}
m_pDialog->ShowWindow(SW_SHOW);
}
// Delete the dialog once done
delete m_pDialog;
Solution 2
Use CDialog::Create and then use CDialog::ShowWindow. You now have a modeless dialog box.
Solution 3
You can call CDialog::Create
and CWnd::ShowWindow
like the others have suggested.
Also, keep in mind your dialog will be destroyed right after its creation if it is stored in a local variable.
Solution 4
In this case I find it most convenient to let it self-delete itself to handle the cleanup.
Often it's considered bad form to make "implicit" memory freeing from within a class, and not by what it created it, but I usually make exceptions for modeless dialog boxes.
That is;
Calling code:
#include "MyDialog.h"
void CMyApp::OpenDialog()
{
CMyDialog* pDlg = new CMyDialog(this);
if (pDlg->Create(IDD_MYDIALOG, this))
pDlg->ShowWindow(SW_SHOWNORMAL);
else
delete pDlg;
}
Dialog code:
void CMapBasicDlg::OnDestroy()
{
CDialog::OnDestroy();
delete this; // Shown as non-modal, we'll clean up ourselves
}
Solution 5
You need to call CDialog::Create
instead. You will need to call DestroyWindow
when you are finished with the dialog. You might also need to pass dialog messages onto the object but I can't remember if MFC handles this for you or not.
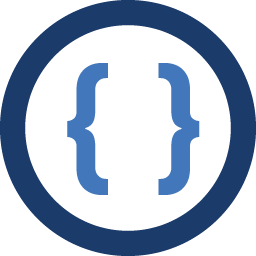
Admin
Updated on August 13, 2020Comments
-
Admin over 3 years
Can someone tell me how I could create a Non Modal Dialog in MFC's Visual c++ 6.0 and show it? I wrote this code:
CDialog dialog; if (dialog.init(initialization values...)) dialog.DoModal();
But it blocks my application from showing the dialog. I dont know if there exists any method or other way to do it.
Thanks