How to display a number with always 2 decimal points using BigDecimal?
Solution 1
BigDecimal is immutable, any operation on it including setScale(2, BigDecimal.ROUND_HALF_UP) produces a new BigDecimal. Correct code should be
BigDecimal bd = new BigDecimal(1);
bd.setScale(2, BigDecimal.ROUND_HALF_UP); // this does change bd
bd = bd.setScale(2, BigDecimal.ROUND_HALF_UP);
System.out.println(bd);
output
1.00
Note - Since Java 9 BigDecimal.ROUND_HALF_UP
has been deprecated and you should now use RoundingMode.ROUND_HALF_UP
.
Solution 2
you can use the round up format
BigDecimal bd = new BigDecimal(2.22222);
System.out.println(bd.setScale(2,BigDecimal.ROUND_UP));
Hope this help you.
Solution 3
To format numbers in JAVA you can use:
System.out.printf("%1$.2f", d);
where d is your variable or number
or
DecimalFormat f = new DecimalFormat("##.00"); // this will helps you to always keeps in two decimal places
System.out.println(f.format(d));
Solution 4
You need to use something like NumberFormat
with appropriate locale to format
NumberFormat.getCurrencyInstance().format(bigDecimal);
Solution 5
BigDecimal.setScale would work.
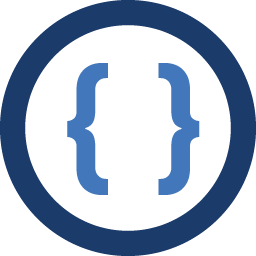
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am using BigDecimal to get some price values. Requirement is something like this, what ever the value we fetch from database, the displayed valued should have 2 decimal points.
Eg:
fetched value is 1 - should be displayed as 1.00
fetched value is 1.7823 - should be displayed as 1.78I am using
setScale(2, BigDecimal.ROUND_HALF_UP)
but still some places, if the data from DB is a whole number then the same is being displayed !!I mean if the value is 0 from DB its displayed as 0 only. I want that to be displayed as 0.00
Thanks