How to display an image which is in bytes to JSP page using HTML tags?
Solution 1
If the image is not too big, you can do it as follows.
<img src="data:image/jpg;base64,iVBORw0KGgoAAAANS..." />
Where iVBORw0KGgoAAAANS
... is the Base64 encoded bytes.
Base64 encoding can be done with a library, like Ostermiller Java Utilities' Base64 Java Library or org.apache.commons.codec.binary.Base64.
Solution 2
Unless you use a "data" URI (useful for small images), the browser will make two requests: one for the HTML and one for the image. You need to be able to output an img
tag which includes enough information to let you respond to the subsequent request for the image with the data in your ByteArrayOutputStream
.
Depending on how you got that JPEG file and how your server scales out, that might involve writing the image to disk, caching it in memory, regenerating it, or any combination of these.
If you can delay the image generation until the browser requests the actual image in the first place, that's pretty ideal. That may involve putting extra parameters in the URL for the image - such as points on a graph, or the size of thumbnail to generate, or whatever your image is.
If you're new to both JSP and HTML, I strongly recommend you concentrate on the HTML side first. Work out what you need to serve and what the browser will do before you work out how to serve it dynamically. Start with static pages and files for the HTML and images, and then work out how to generate them instead.
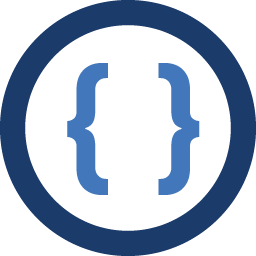
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
I have ByteArrayOutputStream which contains a JPEG image in bytes. My requirement is to display that image in a JSP page (to display the image in the frontend using HTML tags). How do I do that?
I have referred the
BufferedImage
class, but it is confusing for me because I am new to this. -
Prabhu R almost 15 yearsthis method inlines the image into the html that is being rendered which can also increase the file size
-
Admin almost 15 yearsMy image is very small only.. Its a barcode image of size width="166" height="44".. In the above, how do i get image from ByteArrayOutputStream?
-
Jon Skeet almost 15 years166x44 isn't exactly "very small" (usually data URIs are used for really tiny images) but with a bar code you may get away with it. Basically you need to base64 encode the data. That's a separate, very specific question - which has probably already been covered elsewhere on Stack Overflow.
-
Valentin Rocher almost 15 yearsFrom a ByteArrayInputStream, and using Commons : List aList = new ArrayList<Byte>(); int cByte = bais.read(); while (cByte != null) { aList.add(cByte); cByte = bais.read(); } byte[] result = Base64.encode(aList.toArray()); String imageData = new String(result) And you can use imageData.