How to display error message as a modal
11,944
You could try the following solution:
- Instead of using
ViewBag
useTempData["sErrMsg"]
- usingTempData
retains the value accross the current and the subsequent HTTP request. - In the controller action - set
TempData["sErrMsg"]
to the required error message if a specific condition is met. - In the View - check if the
TempData["sErrMsg"]
has a value (contains an error) and if it does useHtml.RenderPartial("ErrorMessageView", TempData["sErrMsg"]);
to render the partial view as a bootstrap modal popup.
Controller:
public class ProductController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult Save(string name)
{
if(String.IsNullOrEmpty(name))
TempData["sErrMsg"] = "Product name cannot be empty";
return View("Index");
}
public PartialViewResult ShowError(String sErrorMessage)
{
return PartialView("ErrorMessageView");
}
}
Index View:
@using (Html.BeginForm("Save", "Product", FormMethod.Post, new { id = "myform" }))
{
@Html.TextBox("name", null, new { style = "width:500px;" })
<input type="submit" value="Add" />
}
@if (TempData["sErrMsg"] != null)
{
Html.RenderPartial("ErrorMessageView", TempData["sErrMsg"]);
}
Partial Error View:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.3/jquery.min.js"></script>
<script src="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script type="text/javascript">
$(function () {
$('.modal').modal('show');
});
</script>
<div class="modal fade" tabindex="-1" role="dialog">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title">Error</h4>
</div>
<div class="modal-body">
@Model
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary">Save changes</button>
</div>
</div>
</div>
</div>
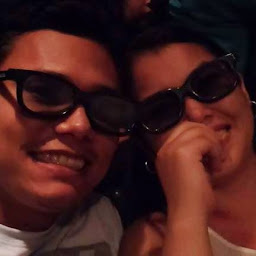
Author by
Alex Fariñas
Updated on June 04, 2022Comments
-
Alex Fariñas almost 2 years
I'm trying to display a error massage as a modal boostrap... the message is sent correctly but the partial view load as common page... (no modal)
I need your help to solve this.. :)
return RedirectToAction("ShowError", new { sErrorMessage = "Error Message" });
This is my ActionResult 'ShowError'
public ActionResult ShowError(String sErrorMessage) { ViewBag.sErrMssg = sErrorMessage; return PartialView("ErrorMessageView"); }
My PartialView 'ErrorMessageView'
<div class="modal fade" tabindex="-1" role="dialog"> <div class="modal-dialog" role="document"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button> <h4 class="modal-title">Error</h4> </div> <div class="modal-body"> @ViewBag.sErrMssg </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> <button type="button" class="btn btn-primary">Save changes</button> </div> </div><!-- /.modal-content --> </div><!-- /.modal-dialog -->