How to display values of an ArrayList that is defined in the JSP itself, using JSTL
20,759
Solution 1
You need to put the array in the request. Do this right after the last fruits.add() call.
<%= request.setAttribute( "fruits", fruits ); %>
Solution 2
add pageContext.setAttribute("fruits", fruits);
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ page import="java.util.ArrayList" %>
<%
ArrayList<String> fruits = new ArrayList<String>();
fruits.add("Orange");
fruits.add("Apple");
pageContext.setAttribute("fruits", fruits);
%>
<html>
<head>
<title>JSTL</title>
</head>
<body>
<c:forEach var="fruit" items="${fruits}">
<c:out value="${fruit}" />
</c:forEach>
</body>
</html>
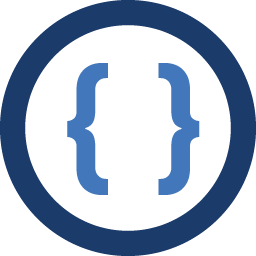
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have an ArrayList that is defined in a scriptlet in a JSP. In the body section, I want to display the items using a JSTL forEach loop.
After going through tutorials like this one, I have written the following code:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <%@ page import="java.util.ArrayList" %> <% ArrayList<String> fruits = new ArrayList<String>(); fruits.add("Orange"); fruits.add("Apple"); %> <html> <head> <title>JSTL</title> </head> <body> <c:forEach var="fruit" items="${fruits}"> <c:out value="${fruit}" /> </c:forEach> </body> </html>
But I am getting a blank page. Where am I going wrong in the above code?
All tutorials that I could find seem to define an ArrayList of beans in the servlet and pass them to the JSP through the
request
. In the forEach loop, they usec:out
and${bean.prop}
to print it. I haven't tried them as such. I wanted to do something much simpler, but can't seem to get this code to work.