How to do a https request with proxy
There doesn't appear to be anything wrong with your Client.Transport.Proxy
configuration. Both attempted approaches (ProxyURL
and ProxyFromEnvironment
) should work fine to specify a forward proxy.
Relevant question: How to program Go to use a proxy?
Something to look out for, however unlikely, is that subsequent client-side calls to not somehow bypass the use of WriteProxy and instead call Write directly -- since this would defeat the proxy specification.
Given the information provided here, "Bad Request" likely indicates that you are reaching the proxy, but that it is not working correctly as a generic HTTP/HTTPS forward proxy, which is what Client.Transport.Proxy
would specify.
Maybe some clarity would be added here: How to use Nginx as a HTTP/HTTPS proxy server?. In summary, NGINX is not normally used as a forward proxy, so maybe this isn't what you are trying to do.
If you are trying to use NGINX as a reverse proxy, then configuration is a server-side concern and your clients do not need special proxy configuration -- just change your request URL to point to the proxy.
Side note: If not otherwise specified, &http.Client{}
will use DefaultTransport
which automatically includes ProxyFromEnvironment
, so the extra proxy configuration is only required if you are constructing a non-default Transport (as is done in the question in order to specify InsecureSkipVerify
) or you want to have an application-specific proxy confuration. And, to repeat, client-side proxy configuration is only needed when using a forward proxy.
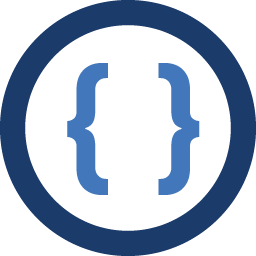
Admin
Updated on July 06, 2022Comments
-
Admin almost 2 years
I am able to do a http or https client request without setting up a proxy,
enter code here tr := &http.Transport{ TLSClientConfig: &tls.Config{InsecureSkipVerify: true}, } client := &http.Client{} client.Transport = tr request, err := http.NewRequest("HEAD", "http://www.???.com", nil) request.Header.Set("User-Agent", "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_11_6) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36") resp, err := client.Do(request) if err != nil { log.Fatalln(err) return } defer resp.Body.Close() httpcode = resp.Status
This script is working ok, I get a 200 ok when I request https url, but if I set up proxy , the script:
proxyString := "https://47.91.179.xxx:443" proxyUrl, _ := url.Parse(proxyString) tr := &http.Transport{ Proxy: http.ProxyURL(proxyUrl), TLSClientConfig: &tls.Config{InsecureSkipVerify: true}, } client := &http.Client{} client.Transport = tr ....................
I always get "Bad request", I read docs: https://golang.org/pkg/net/http/:
........... Starting with Go 1.6, the http package has transparent support for the HTTP/2 protocol when using HTTPS. Programs that must disable HTTP/2 can do so by setting Transport.TLSNextProto (for clients) or Server.TLSNextProto (for servers) to a non-nil, empty map. Alternatively, the following GODEBUG environment variables are currently supported: ..............
So, I tried to stop http2:
tr := &http.Transport{ Proxy: http.ProxyURL(proxyUrl), //Proxy: http.ProxyFromEnvironment, TLSClientConfig: &tls.Config{InsecureSkipVerify: true}, TLSNextProto: make(map[string]func(authority string, c *tls.Conn) http.RoundTripper), }
same problem, "Bad request". I tried os.Setenv("HTTPS_PROXY", "47.91.???.???:443"), It is the same result. This is logged in server:
{S;}220.255.95.68{S;}-{S;}07/Mar/2017:17:01:47 +0800{S;}CONNECT www.panpacific.com:443 HTTP/1.1{S;}400{S;}173{S;}340{S;}-{S;}-{S;}-{S;}-{S;}-{S;}www.panpacific.com
So, Does golang support https client request via proxy? How do I get correct results?
-
Brent Bradburn over 4 yearsForward HTTP proxy: How exactly does a proxy work?