How to download PDF File Generated from TCPDF (PHP) using AJAX (jQuery)?
Solution 1
If the problem is that you are not getting the browser's download dialog for the PDF, then the solution is to do it this way:
First, redirect the browser (using window.location
as the other answers say) to navigate to a special controller action in your application, e.g. with this url: http://your.application.com/download/pdf/filename.pdf
.
Implement the action referenced in the URL like this:
public function actionPdf() {
header('Content-Type: application/pdf');
header('Content-Disposition: attachment; filename="filename.pdf";');
header('Content-Length: '.filesize('path/to/pdf'));
readfile('path/to/pdf');
Yii::app()->end();
}
This will cause the browser to download the file.
Solution 2
You need to save the PDF to somewhere on your server and then issue window.location = '/url/to/pdf-you-just-saved.pdf';
from your javascript. The users browser will then prompt them to download the PDF file.
Solution 3
in tcpdf , just pass this argument the Output method:
$pdf->Output('yourfilename.pdf', 'D');
that's all
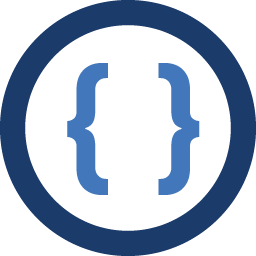
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am using Yii Framework, TCPDF and jQuery to generate a pdf.
The pdf is generated by inputing in a form and submitting it using ajax.
The pdf is created but here is the problem when it returns to the client, it down not download.
here is the php code
$pdf->Output('Folder Label.pdf','D');
the jQuery on success function has
success: function(data) { window.open(data); }
Which i got from this site.
Can you please help
-
Treffynnon about 13 yearsSee developer.mozilla.org/en/DOM/window.location Specifically "newLocation is a Location object or a string, specifying the URL to navigate to." List the browsers that have an issue with
window.location = '';
please. -
Joseph Lust about 13 years@Treffynnon - Simple: Internet Explorer. stackoverflow.com/questions/1939332/window-location-problem
-
Treffynnon about 13 yearsI have never had an issue with this in IE7+. However I have not supported IE6 for a long time and neither do my clients or their users. ie6countdown.com
-
Joseph Lust about 13 years@Treffynnon If only all developers had such freedom. Frankly IE6 is a gaffe factory. You should count yourself lucky.
-
Tyler over 6 yearsThe OP specifies AJAX. AJAX is generally used to bring in content without reloading the page - in otherwords, there is probably content already outputted. TCPDF will (as I have just seen in my situation) generate an error when you try to output with content already outputted:
TCPDF ERROR: Some data has already been output, can't send PDF file