How to enable and disable vibration mode programmatically
Solution 1
We can enable and disable the silent mode programmatically by using AudioManager:
AudioManager audioManager = (AudioManager) getSystemService(AUDIO_SERVICE);
for setting silent mode :
audioManager.setRingerMode(AudioManager.RINGER_MODE_SILENT);
For normal mode :
audioManager.setRingerMode(AudioManager.RINGER_MODE_NORMAL);
Solution 2
First of all use this permission in AndroidManifest.xml
<uses-permission android:name="android.permission.VIBRATE"/>
Now
public void startVibrate(View v) {
long pattern[] = { 0, 100, 200, 300, 400 };
vibrator = (Vibrator) getSystemService(Context.VIBRATOR_SERVICE);
vibrator.vibrate(pattern, 0);
}
public void stopVibrate(View v) {
vibrator.cancel();
}
Vibrate pattern public abstract void vibrate (long[] pattern, int repeat) Pattern for vibration is nothing but an array of duration's to turn ON and OFF the vibrator in milliseconds. The first value indicates the number of milliseconds to wait before turning the vibrator ON. The next value indicates the number of milliseconds for which to keep the vibrator on before turning it off. Subsequent values, alternates between ON and OFF.
long pattern[]={0,100,200,300,400};
If you feel not to have repeats, just pass -1 for 'repeat'. To repeat patterns, just pass the index from where u wanted to start. I wanted to start from 0'th index and hence I am passing 0 to 'repeat'.
vibrator.vibrate(pattern, 0);
Solution 3
myAudioManager.setVibrateSetting();
This method was deprecated in API level 16.
you can use this one:
audioManager.setRingerMode(AudioManager.RINGER_MODE_SILENT)
RINGER_MODE_SILENT : will mute the volume and will not vibrate.
RINGER_MODE_VIBRATE: will mute the volume and vibrate.
RINGER_MODE_NORMAL: will be audible and may vibrate according to user settings.
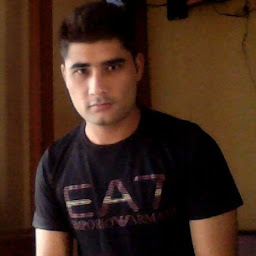
Arun kumar
Updated on June 14, 2022Comments
-
Arun kumar almost 2 years
I need to enable and disable the vibration mode of mobile when user turns off and turns on the switch button .
I have tried the code below, but it's not working:
AudioManager myAudioManager; myAudioManager = (AudioManager)getSystemService(Context.AUDIO_SERVICE); Toast.makeText(this, "in setting "+(myAudioManager.getMode()==AudioManager.RINGER_MODE_VIBRATE),1).show(); if(myAudioManager.getMode()==AudioManager.RINGER_MODE_VIBRATE) { //myAudioManager.setRingerMode(AudioManager.RINGER_MODE_NORMAL); myAudioManager.setVibrateSetting(AudioManager.VIBRATE_TYPE_RINGER, AudioManager.VIBRATE_SETTING_OFF); } else { //myAudioManager.setRingerMode(AudioManager.RINGER_MODE_VIBRATE); myAudioManager.setVibrateSetting(AudioManager.VIBRATE_TYPE_RINGER, AudioManager.VIBRATE_SETTING_ON); }