How to enble/disable a button in TypeScript 1.5?
Solution 1
There should be a cleaner way to do this:
var element = <HTMLInputElement> document.getElementById("btnExcel");
element.disabled = true;
Or if you prefer a one liner:
(<HTMLInputElement> document.getElementById("btnExcel")).disabled = true;
It seems getElementById
and the like should be a generic method and accept a type parameter. That said, a generic method wouldn't give you anything that typecasting doesn't.
Solution 2
I'm using TypeScript v 3.7.something. In here
(document.getElementById('button') as HTMLInputElement).disabled = false;
is prefered. (guided by @Martin's answer)
Solution 3
I'm using "typescript": "~3.8.3" in Angular 9. To disable a button successfully try:
let button = <HTMLButtonElement> document.getElementById('confirmBtn');
button.disabled = true;
Solution 4
Problem solved by using <HTMLInputElement>
Just try this..
var previous = <HTMLInputElement>document.getElementById('previous');
previous.disabled = true;
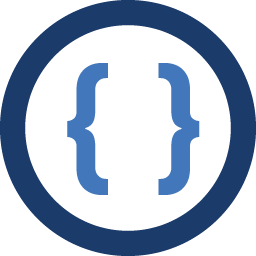
Admin
Updated on March 26, 2021Comments
-
Admin about 3 years
Using VS 2013. After having installed TypeScript 1.5 and following the question/suggestion to upgrade:
"Your project uses a version of TypeScript older than the version currently installed with Visual Studio. You may get errors if you try to build your project. Would you like us to upgrade the TypeScriptToolsVersion in your project file so you don't see this warning again?"
I got a bunch of errors like:
Error 39 Build: Property 'disabled' does not exist on type 'HTMLElement'.
on statements like:
document.getElementById("btnExcel").disabled = false;
with 'disabled' curly underlined.
On https://github.com/Microsoft/TypeScript/wiki/Breaking-Changes referring to version 1.5 it says: "Properties on resize, disabled, uniqueID, removeNode, fireEvent, currentStyle, runtimeStyle are removed from type HTMLElement"
Now I rephrased those "erroneous" statements like this:
document.getElementById("btnExcel").setAttribute('disabled', 'disabled');
which to me looks weird.
Can this be expressed more elegantly in a typesafe way in TypeScript 1.5? Can you give examples for both: enabling and disabling?
Thanks for any help!
-
Ziggler over 5 yearsIn typescript I am unable to find disabled property,,,,
-
MadHenchbot almost 5 years@Ziggler Make sure you cast the element first, or disabled won't be an option. <HTMLInputElement>
-
Martin almost 5 years@ziggler yep, that's what the parens do, cast the element so that the property can be selected.
-
PhillipJacobs over 4 yearsThanks so much @Martin 🔥👨🏽💻