How to encode a URL in WinForms?
22,498
Solution 1
If you need to URL-encode data for a querystring, you can use either Uri.EscapeDataString or, if you don't mind referencing System.Web, HttpUtility.UrlEncode:
var rawString = @"this & that";
var uriEncoded = Uri.EscapeDataString(rawString);
var httpUtilityEncoded = HttpUtility.UrlEncode(rawString);
They're very similar but can produce subtly different results in the way special characters, like spaces, are encoded:
Console.WriteLine(uriEncoded);
// uriEncoded = "this%20%26%20that"
Console.WriteLine(httpUtilityEncoded);
// httpUtilityEncoded = "this+%26+that"
Solution 2
Solution 3
Did you tried with:
var url = System.Net.WebUtility.UrlEncode(string);
You don't need a dependency on System.Web and you can use it in PCL, I used it in my Xamarin forms project.
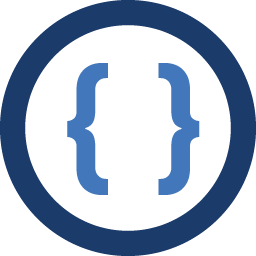
Author by
Admin
Updated on September 15, 2020Comments
-
Admin over 3 years
I'm creating a Windows application and I need to pass an encoded URL. But I'm not sure how to encode it in WinForms C#?
-
Dan about 6 yearsUPDATE: If you don't have access to HttpUtility class from System.Web that means its replaced with WebUtility. In case if you creating
UriBuilder
class where you append your encoded string withUriBuilder.AppendFormat
encoded value will be in Builder.Uri.AbsoluteUri field. If you say .ToString() on UriBuilder class, not all characters will be encoded. On this, I preferably think on umlauts, which you can find in the German language for example. -
ZeroWorks almost 5 yearsYou have to mind about length of the string to URL-encode... if it's larger than 32768 you must use
HttpUtility.UrlEncode
.