How to end a while Loop via user input
26,993
Solution 1
You have to assign repeat
in your while
-loop so it becomes false
if the user says yes
:
repeat = !input.equalsIgnoreCase("yes");
Solution 2
You just need to set repeat
to true or false based on user input. So in the end, compare input
with yes or no. Something like this would work for you :
if ("yes".equals(input))
repeat = true; // This would continue the loop
else
repeat = false; // This would break the infinite while loop
Solution 3
you also if you want your code to be more systematic , go and search about the interrupt , specially thread interrupt , these answers above is correct , find the more organic code and implement it
Solution 4
boolean repeat = true;
// Create a Scanner object for keyboard input.
Scanner keyboard = new Scanner(System.in);
while (repeat)
{
-----------------------
-------------------------
System.out.println("Do you want to continue:");
repeat = keyboard.nextBoolean();
}
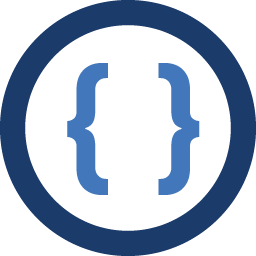
Author by
Admin
Updated on September 24, 2020Comments
-
Admin over 3 years
package cst150zzhw4_worst; import java.util.Scanner; public class CST150zzHW4_worst { public static void main(String[] args) { //Initialize Variables double length; // length of room double width; // Width of room double price_per_sqyd; // Total carpet needed price double price_for_padding; // Price for padding double price_for_installation; // Price for installation String input; // User's input to stop or reset program double final_price; // The actual final price boolean repeat = true; // Create a Scanner object for keyboard input. Scanner keyboard = new Scanner(System.in); while (repeat) { //User Input System.out.println("\n" +"What is the length of the room?: "); length = keyboard.nextInt(); System.out.println("What is the width of the room?: "); width = keyboard.nextInt(); System.out.println("What is the price of the carpet per square yard?: "); price_per_sqyd = keyboard.nextDouble(); System.out.println("What is the price for the padding?: "); price_for_padding = keyboard.nextDouble(); System.out.println("What is the price of the installation?: "); price_for_installation = keyboard.nextDouble(); final_price = (price_for_padding + price_for_installation + price_per_sqyd)*((width*length)/9); keyboard.nextLine(); //Skip the newline System.out.println("The possible total price to install the carpet will be $" + final_price + "\n" + "Type 'yes' or 'no' if this is correct: "); input = keyboard.nextLine(); } } }
How would I make it so when the user says yes the program stop and if the user says no then the program just repeats? I don't know why I'm having so much trouble. I've searched for well over 4 hours. I am only supposed to use a while loop, I think.