How to execute insert query using Entity Framework
19,805
Solution 1
You missing the closing ) at the end of your INSERT.
It should be:
Context.database.ExecuteSqlCommand("Insert into tableName Values({0},{1},{2})", param1, param2, param3)
Alternatively, you could use the SqlParameter class:
Context.Database.ExecuteSqlCommand(
"Insert into tableName Values(@id, @firstName, @lastName)",
new SqlParameter("id", id),
new SqlParameter("firstName", firstName),
new SqlParameter("lastName", lastName)
);
Solution 2
Apart from the obvious question why you are writing SQL, it seems you are missing a closing parenthesis, after the third {2}
parameter:
Contex.database.ExecuteSQLCOmmand(@"Insert into tableName Values({0},{1},{2})",param1,param2,param3);
Then it is also a good practise to specify column names, like so:
Contex.database.ExecuteSQLCOmmand(@"Insert into tableName (col1, col2, col3) Values({0},{1},{2})",param1,param2,param3);
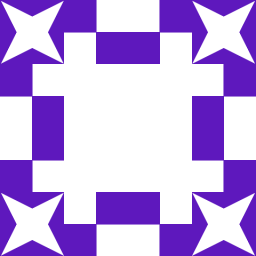
Author by
Ajay George
Updated on June 09, 2022Comments
-
Ajay George 12 months
I am trying to execute a insert query using Entity Framework.
I have something like this:
Context.database.ExecuteSqlCommand("Insert into tableName Values({0},{1},{2}", param1, param2, param3)
but this is throwing an error
incorrect syntax near '@p1'
What is this '@p1' ?