How to find if two numbers are consecutive numbers in gray code sequence
Solution 1
I've had to solve this question in an interview as well. One of the conditions for the two values to be a gray code sequence is that their values only differ by 1 bit. Here is a solution to this problem:
def isGrayCode(num1, num2):
differences = 0
while (num1 > 0 or num2 > 0):
if ((num1 & 1) != (num2 & 1)):
differences++
num1 >>= 1
num2 >>= 1
return differences == 1
Solution 2
Actually, several of the other answers seem wrong: it's true that two binary reflected Gray code neighbours differ by only one bit (I assume that by « the » Gray code sequence, you mean the original binary reflected Gray code sequence as described by Frank Gray). However, that does not mean that two Gray codes differing by one bit are neighbours (a => b
does not mean that b => a
). For example, the Gray codes 1000 and 1010 differ by only one bit but are not neighbours (1000 and 1010 are respectively 15 and 12 in decimal).
If you want to know whether two Gray codes a
and b
are neighbours, you have to check whether previous(a) = b OR next(a) = b
. For a given Gray code, you get one neighbour by flipping the rightmost bit and the other neighbour bit by flipping the bit at the left of the rightmost set bit. For the Gray code 1010, the neighbours are 1011 and 1110 (1000 is not one of them).
Whether you get the previous or the next neighbour by flipping one of these bits actually depends on the parity of the Gray code. However, since we want both neighbours, we don't have to check for parity. The following pseudo-code should tell you whether two Gray codes are neighbours (using C-like bitwise operations):
function are_gray_neighbours(a: gray, b: gray) -> boolean
return b = a ^ 1 OR
b = a ^ ((a & -a) << 1)
end
Bit trick above: a & -a
isolates the rigthmost set bit in a number. We shift that bit by one position to the left to get the bit we need to flip.
Solution 3
Assumptions: Inputs a and b are grey code sequences in binary reflected gray code. i.e a's and b's bit encoding is binary gray code representations.
#convert from greycode bits into regular binary bits
def gTob(num): #num is binary graycode
mask = num >> 1
while mask!=0:
num = num^mask
mask >>= 1
return num; #num is converted
#check if a and b are consecutive gray code encodings
def areGrayNeighbors(a,b):
return abs(gTob(a) - gTob(b)) == 1
Few Test cases:
- areGrayNeighbors(9,11) --> True (since (1001, 1011) differ in only one bit and are consecutive numbers in decimal representation)
- areGrayNeighbors(9,10) --> False
- areGrayNeighbors(14,10) --> True
References: method gTob() used above is from rodrigo in this post The neighbors in Gray code
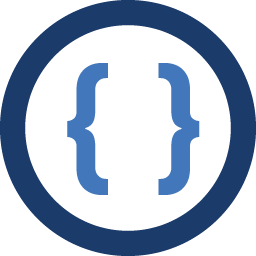
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I am trying to come up with a solution to the problem that given two numbers, find if they are the consecutive numbers in the gray code sequence i.e., if they are gray code neighbors assuming that the gray code sequence is not mentioned.
I searched on various forums but couldn't get the right answer. It would be great if you can provide a solution for this.
My attempt to the problem - Convert two integers to binary and add the digits in both the numbers separately and find the difference between the sum of the digits in two numbers. If the difference is one then they are gray code neighbors.
But I feel this wont work for all cases. Any help is highly appreciated. Thanks a lot in advance!!!
-
Sameer Sawla over 9 yearsAwesome solution. This is best one out of all and the correct one too. Nice job man.
-
Exectron about 9 yearsThis answer is wrong. See Morwenn's answer.
-
Morwenn about 9 years@DavidJones « [...] it's true that two Gray code neighbours differ by only one bit. However, that does not mean that two Gray codes differing by one bit are neighbours (
a => b
does not mean thatb => a
). » -
iRuth about 9 yearsPlease add a little explanation as to why you think this answers the OP's question.
-
greybeard about 9 yearsDid you try to read and understand the accepted answer and Morwenn's, and why they are rated differently?
-
Aswin about 9 yearsWhat's the down vote for? I've clearly mentioned that this method only checks the first criteria. During interviews at tech companies, I've been asked to write the code for this specific task and they were not interested in the exact Gray Code adjacency test.
-
Di Wu over 8 yearsBased on the accepted answer at math.stackexchange.com/questions/965168/… , your pseudo-code is not able to go through all the neighbour possibilities. Example of two Gray Code sequences of the same length: [000,001,011,010,110,111,101,100], [000,001,011,111,101,100,110,010]. The code 000 could have more than two legit neighbours.
-
Morwenn over 8 years@DiWu Of course, my answer assumes a binary reflected Gray code sequence, which is generally the one people are saking about when nothing is specified.
-
greybeard over 3 yearsThis does not seem to differ from David.Jones's erroneous accepted answer.