How to force users to update the app using react native
Solution 1
You can check for the App Store / Play Store version of your app by using this library react-native-appstore-version-checker.
In expo app you can get the current bundle version using Constants.nativeAppVersion
. docs.
Now in your root react native component, you can add an event listener to detect app state change. Every time the app transitions from background to foreground, you can run your logic to determine the current version and the latest version and prompt the user to update the app.
import { AppState } from 'react-native';
class Root extends Component {
componentDidMount() {
AppState.addEventListener('change', this._handleAppStateChange);
}
_handleAppStateChange = (nextState) => {
if (nextState === 'active') {
/**
Add code to check for the remote app version.
Compare it with the local version. If they differ, i.e.,
(remote version) !== (local version), then you can show a screen,
with some UI asking for the user to update. (You can probably show
a button, which on press takes the user directly to the store)
*/
}
}
componentWillUnmount() {
AppState.removeEventListener('change', this._handleAppStateChange);
}
}
Solution 2
import VersionCheck from 'react-native-version-check';
i have used version check lib for this purpose and approach i used is below. if version is lower i'm opening a modal on which an update button appears, and that button redirects to app store/google play
componentDidMount() {
this.checkAppUpdate();
}
checkAppUpdate() {
VersionCheck.needUpdate().then(res => {
if (res.isNeeded) {
setTimeout(() => {
this.setState({openModal: true});
});
}
});
}
updateApp = () => {
VersionCheck.getStoreUrl({
appID: 'com.showassist.showassist',
appName,
})
.then(url => {
Linking.canOpenURL(url)
.then(supported => {
if (!supported) {
} else {
return Linking.openURL(url);
}
})
.catch(err => console.error('An error occurred', err));
})
.catch(err => {
console.log(`error is: ${err}`);
});
};
Related videos on Youtube
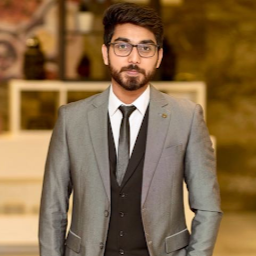
Arham Shahzad
Updated on June 11, 2022Comments
-
Arham Shahzad almost 2 years
I have updated my app on app and play store and I want to force my app users to update the new version of app in App store and playstore.
-
Nate about 3 yearsMine crashes after build but works well in development. Did you experience same?
-
Hp Sharma about 3 yearsNo, I did not face this issue.
-
Birender Pathania about 3 yearsI am using github.com/kimxogus/react-native-version-check for the verison check but its not working its returning me undefined: VersionCheck.needUpdate().then(res => { if (res.isNeeded) { setTimeout(() => { this.setState({openModal: true}); }); } });
-
Syed Amir Ali about 3 yearsversion check doesn't work on Emulators you need to check this on physical device @BirenderPathania
-
Rahul Khurana over 2 years@SyedAmirAli I am testing on a real device. It is giving me
[TypeError: Invalid Version: 1.03.0]
error