How to generate soap request xml from wsdl file in java
Solution 1
You can use the open-source Membrane SOA library ([http://www.membrane-soa.org/soa-model-doc/1.4/java-api/create-soap-request-template.htm ]) to generate XMLs for each operation defined in the WSDL:
public void createTemplates(String url){
WSDLParser parser = new WSDLParser();
Definitions wsdl = parser.parse(url);
StringWriter writer = new StringWriter();
SOARequestCreator creator = new SOARequestCreator();
creator.setBuilder(new MarkupBuilder(writer));
creator.setDefinitions(wsdl);
for (Service service : wsdl.getServices()) {
for (Port port : service.getPorts()) {
Binding binding = port.getBinding();
PortType portType = binding.getPortType();
for (Operation op : portType.getOperations()) {
creator.setCreator(new RequestTemplateCreator());
creator.createRequest(port.getName(), op.getName(), binding.getName());
System.out.println(writer);
writer.getBuffer().setLength(0);
}
}
}
Solution 2
Soap UI also provide Java Api for creating request and response xml from WSDL.
public static void main(String[] args) throws Exception {
WsdlProject project = new WsdlProject();
WsdlInterface[] wsdls = WsdlImporter.importWsdl(project, "http://localhost:8080/Service?wsdl");
WsdlInterface wsdl = wsdls[0];
for (Operation operation : wsdl.getOperationList()) {
WsdlOperation wsdlOperation = (WsdlOperation) operation;
System.out.println("Request:\n"+wsdlOperation.createRequest(true));
System.out.println("\nResponse:\n"+wsdlOperation.createResponse(true));
}
}
Developer's corner of Soap UI has nice pointers for integrating with soap UI Api.
Solution 3
Have a look at AXIS
http://axis.apache.org/axis2/java/core/
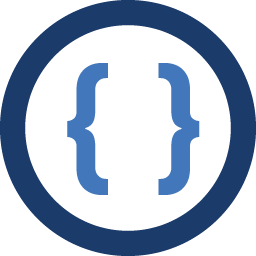
Admin
Updated on January 29, 2020Comments
-
Admin over 4 years
I am looking for some java opensource api for generating soap request xml file by passing wsdl_URL and operation name as parameters. Actually soapUI is doing this and I tried to go through the soapUI source code, but I am not able to understand the whole code to get my task done.
Is there any java api available to do this (apache or something)?
I spent couple of days in the net and didn't see any result.
If any body has any idea please help me.
Thanks in advance.