How to get a category listing from Magento?
Solution 1
From code found in an SEO related class (Mage_Catalog_Block_Seo_Sitemap_Category)
$helper = Mage::helper('catalog/category');
$collection = $helper->getStoreCategories('name', true, false);
$array = $helper->getStoreCategories('name', false, false);
Try to forget that it's a database that's powering your store, and instead concentrate on using the objects that the Magento system provides.
For example, I had no no idea how to get a list of categories. However, I grepped through the Mage codebase with
grep -i -r -E 'class.+?category'
Which returned a list of around 30 classes. Scrolling through those, it was relatively easy to guess which objects might have methods or need to make method calls that would grab the categories.
Solution 2
Hey something like this might help you, I've adapted it slightly to answer your question more specifically.
$h3h3=Mage::getModel('catalog/category')->load(5); // YOU NEED TO CHANGE 5 TO THE ID OF YOUR CATEGORY
$h3h3=$h3h3->getProductCollection();
foreach($h3h3->getAllIds() as $lol)
{
$_product=Mage::getModel('catalog/product')->load($lol);
print $_product->getName()."<br/>";
}
Solution 3
I adapted this from Paul Whipp's website:
SELECT e.entity_id AS 'entity_id', vn.value AS 'name'
FROM catalog_category_entity e
LEFT JOIN catalog_category_entity_varchar vn
ON e.entity_id = vn.entity_id AND vn.attribute_id = 33
ORDER BY entity_id;
This will provide you with the catalog category IDs.
Solution 4
Here's a quick example
$categories = Mage::getModel('catalog/category')->getCollection()
->addAttributeToSelect('name')
->addAttributeToSelect('url_key')
->addAttributeToSelect('my_attribute')
->setLoadProductCount(true)
->addAttributeToFilter('is_active',array('eq'=>true))
->load();
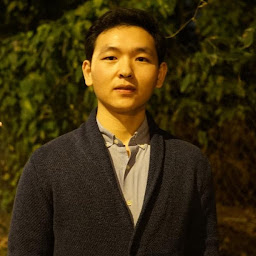
Martin Lee
Updated on September 25, 2020Comments
-
Martin Lee over 3 years
I've recently started to learn the C programming language and therefore started tackling problems on Hackerrank. One of the problems is as follows:
In this problem, you need to print the pattern of the following form containing the numbers from 1 to
n
.4 4 4 4 4 4 4 4 3 3 3 3 3 4 4 3 2 2 2 3 4 4 3 2 1 2 3 4 4 3 2 2 2 3 4 4 3 3 3 3 3 4 4 4 4 4 4 4 4
I saw a programmer on YouTube. He wrote a very short program to solve it:
#include <stdio.h> #include <string.h> #include <math.h> #include <stdlib.h> #define max(x,y) ((x)>(y)?x:y) int main() { int n; scanf("%d", &n); int len = n*2 - 1; for (int i = 0; i < len; i++){ for(int j = 0; j < len; j++){ printf("%d ",max( abs(n -i-1) + 1, abs(n-j-1) +1 )); } printf("\n"); } return 0; }
I already listed all the indexing of the
2n-1 x 2n-1
matrix and tried to observe the relationship. But I can't formulate the relation ofmax( abs(n -i-1) + 1, abs(n-j-1) +1 )
.I just don't get the idea of
max( abs(n -i-1) + 1, abs(n-j-1) +1 )
, why does this line of code get the correct the value of each element in the matrix? -
Devplex almost 15 yearsI'm not sure how you can actually use the resulting collection or array to get the names or url's of categories. That data I need is stored in the "_data:protected" keys of the result. Could you elaborate?
-
Alan Storm almost 15 years
-
Mufaddal almost 12 yearsMy question is , if this is helpful in admin module file, to load category listing?