How to get all the values from appsettings key which starts with specific name and pass this to any array?
56,644
Solution 1
I'd use a little LINQ:
string[] repositoryUrls = ConfigurationManager.AppSettings.AllKeys
.Where(key => key.StartsWith("Service1URL"))
.Select(key => ConfigurationManager.AppSettings[key])
.ToArray();
Solution 2
You are overwriting the array for every iteration
List<string> values = new List<string>();
foreach (string key in ConfigurationManager.AppSettings)
{
if (key.StartsWith("Service1URL"))
{
string value = ConfigurationManager.AppSettings[key];
values.Add(value);
}
}
string[] repositoryUrls = values.ToArray();
Solution 3
I defined a class to hold the variables I am interested in and iterate through the properties and look for something in the app.config to match.
Then I can consume the instance as I wish. Thoughts?
public static ConfigurationSettings SetConfigurationSettings
{
ConfigurationSettings configurationsettings = new ConfigurationSettings();
{
foreach (var prop in configurationsettings.GetType().GetProperties())
{
string property = (prop.Name.ToString());
string value = ConfigurationManager.AppSettings[property];
PropertyInfo propertyInfo = configurationsettings.GetType().GetProperty(prop.Name);
propertyInfo.SetValue(configurationsettings, value, null);
}
}
return configurationsettings;
}
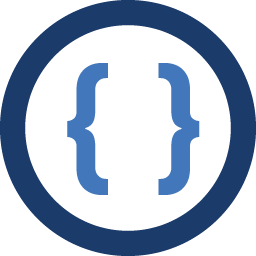
Author by
Admin
Updated on June 13, 2020Comments
-
Admin almost 4 years
In my
web.config
file I have<appSettings> <add key="Service1URL1" value="http://managementService.svc/"/> <add key="Service1URL2" value="http://ManagementsettingsService.svc/HostInstances"/> ....lots of keys like above </appSettings>
I want to get the value of key that starts with
Service1URL
and pass the value tostring[] repositoryUrls = { ... }
in my C# class. How can I achieve this?I tried something like this but couldn't grab the values:
foreach (string key in ConfigurationManager.AppSettings) { if (key.StartsWith("Service1URL")) { string value = ConfigurationManager.AppSettings[key]; } string[] repositoryUrls = { value }; }
Either I am doing it the wrong way or missing something here. Any help would really be appreciated.