how to get background color back to previous color after use of std handle
I'm going to port my Pascal code to C++.. it works I've tested it. The solution is to use GetConsoleScreenBufferInfo
before setting the colours via attributes
.. Then restore the attributes
right after..
#include <windows.h>
#include <stdio.h>
void SetConsoleColour(WORD* Attributes, DWORD Colour)
{
CONSOLE_SCREEN_BUFFER_INFO Info;
HANDLE hStdout = GetStdHandle(STD_OUTPUT_HANDLE);
GetConsoleScreenBufferInfo(hStdout, &Info);
*Attributes = Info.wAttributes;
SetConsoleTextAttribute(hStdout, Colour);
}
void ResetConsoleColour(WORD Attributes)
{
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), Attributes);
}
int main()
{
WORD Attributes = 0;
SetConsoleColour(&Attributes, FOREGROUND_INTENSITY | FOREGROUND_RED);
printf("Foreground change..\n");
ResetConsoleColour(Attributes);
printf("Normal attributes..\n");
SetConsoleColour(&Attributes, BACKGROUND_INTENSITY | BACKGROUND_RED);
printf("Background change..\n");
ResetConsoleColour(Attributes);
printf("Normal attributes..\n");
SetConsoleColour(&Attributes, FOREGROUND_INTENSITY | FOREGROUND_RED);
printf("Mixture");
ResetConsoleColour(Attributes);
printf(" of ");
SetConsoleColour(&Attributes, FOREGROUND_INTENSITY | FOREGROUND_RED | FOREGROUND_GREEN);
printf("both..\n");
ResetConsoleColour(Attributes);
SetConsoleColour(&Attributes, FOREGROUND_INTENSITY | FOREGROUND_RED);
printf("Mixture");
ResetConsoleColour(Attributes);
printf(" of ");
SetConsoleColour(&Attributes, FOREGROUND_INTENSITY | FOREGROUND_RED | FOREGROUND_GREEN);
printf("all ");
ResetConsoleColour(Attributes);
SetConsoleColour(&Attributes, BACKGROUND_INTENSITY | BACKGROUND_BLUE);
printf("three");
ResetConsoleColour(Attributes);
printf(" ");
SetConsoleColour(&Attributes, BACKGROUND_INTENSITY | BACKGROUND_RED | BACKGROUND_BLUE | FOREGROUND_INTENSITY | FOREGROUND_RED | FOREGROUND_GREEN | FOREGROUND_BLUE);
printf("in a single line\n");
ResetConsoleColour(Attributes);
}
You can also do this for the "previous" colour by saving its attributes the same way.. You can write your own function that changes the colour, prints and then resets for you.. You can do this for coloured INPUT as well.. Up to you how you use these..
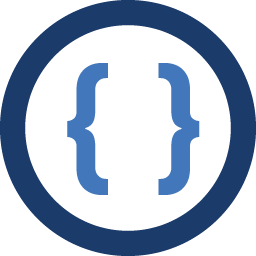
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm using Visual Studio for C++ and we're writing our first code, however I've run into a 'simple' problem.
In the code, I'm using each section as a function in itself, so for the output screen where it says "hit enter", it's calling a function to show that on the screen. However, in the farewell, I changed the system color so that the background would be white and the text black, but the "Hit enter" function still needs to be displayed. It does, but since it uses it's own colors, there's now a line of color where "\t" is in the cout.
How do I get it where it won't do this?
#include <iostream> //Necessary for input/output #include <string> //Necessary for constants #include <Windows.h> //Necessary for colored text using namespace std;
Uh... I've never done this before... but I'll just post the sections seperately.
system("cls"); system("color F0"); cout << "\n\n\n\n\n\n\n\t\tIt was a pleasure spending time with you, " "User" "!\n\n\n"; cout << "\t\t\t\350"; for (int i = 0; i < 31; i++){ cout << "\360"; } cout << "\350\n"; cout << "\t\t\t\272"; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 15); //light grey cout << " \311\315\273\332\304\277\332\304\277\332\302\277\332\277 \302 \302\332\304\277 "; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 240); //Black text white bg cout << "\272\n" "\t\t\t\272"; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 15); //light grey cout << " \272 \313\263 \263\263 \263 \263\263\303\301\277\300\302\331\303\264 "; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 240); //Black text white bg cout << "\272\n" "\t\t\t\272"; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 15); //light grey cout << " \310\315\274\300\304\331\300\304\331\304\301\331\300\304\331 \301 \300\304\331 "; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 240); //Black text white bg cout << "\272\n"; cout << "\t\t\t\350"; for (int i = 0; i < 31; i++){ cout << "\360"; } cout << "\350\n";
And for the Hit enter function
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 7); //light grey cout << "\n\n\n\t\t\t\332"; for (int i = 0; i < 28; i++){ cout << "\304"; } cout << "\277\n\t\t\t\263 "; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 12); // Red cout << "\3 "; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 71); //light grey text with red BG cout << "Please press the Enter"; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 12); // Red cout << " \3 "; SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), 7); //light grey cout << "\263\n\t\t\t\324"; for (int i = 0; i < 28; i++){ cout << "\315"; } cout << "\276";
The "Light grey" area colors the tabs too.... what do I do. Sorry if this is all terrible. New at this.