How to get Current Location(Street,City, etc,..) using gps in Android
19,140
Solution 1
Try this :
public void setCurrentLocation() {
if (UtilityMethods.isGPSEnabled(mContext)) {
if (Build.VERSION.SDK_INT >= 23 &&
ContextCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED &&
ContextCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
requestPermissions(LOCATION_PERMS, LOCATION_REQUEST);
// return;
} else {
getCurrentAddress();
}
} else {
alertbox("Gps Status", "Your Device's GPS is Disable", mContext);
}
}
Using LocationManager
public void getCurrentAddress() {
// Get the location manager
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (locationManager != null) {
try {
if (Build.VERSION.SDK_INT >= 23 && checkSelfPermission(Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && checkSelfPermission(Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// public void requestPermissions(@NonNull String[] permissions, int requestCode)
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String[] permissions,
// int[] grantResults)
// to handle the case where the user grants the permission. See the documentation
// for Activity#requestPermissions for more details.
return;
}
locationManager.requestLocationUpdates(
LocationManager.NETWORK_PROVIDER,
MIN_TIME_BW_UPDATES,
MIN_DISTANCE_CHANGE_FOR_UPDATES, this);
} catch (Exception ex) {
Log.i("msg", "fail to request location update, ignore", ex);
}
if (locationManager != null) {
location = locationManager
.getLastKnownLocation(LocationManager.NETWORK_PROVIDER);
}
Geocoder gcd = new Geocoder(getBaseContext(),
Locale.getDefault());
List<Address> addresses;
try {
addresses = gcd.getFromLocation(location.getLatitude(),
location.getLongitude(), 1);
if (addresses.size() > 0) {
String address = addresses.get(0).getAddressLine(0); // If any additional address line present than only, check with max available address lines by getMaxAddressLineIndex()
String locality = addresses.get(0).getLocality();
String subLocality = addresses.get(0).getSubLocality();
String state = addresses.get(0).getAdminArea();
String country = addresses.get(0).getCountryName();
String postalCode = addresses.get(0).getPostalCode();
String knownName = addresses.get(0).getFeatureName();
if (subLocality != null) {
currentLocation = locality + "," + subLocality;
} else {
currentLocation = locality;
}
current_locality = locality;
}
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(HomeActivity.this, Constants.ToastConstatnts.ERROR_FETCHING_LOCATION, Toast.LENGTH_SHORT).show();
}
}
}
Solution 2
Use fused Location Provider to get current device latitude and longitude.
With the help of latitude and longitude, you can get city name and address.
To get full street name, use getMaxAddressLineIndex().
In onLocationChanged, check whether you are getting current location or not.
Edit:
String strAdd = "";
@Override
public void onLocationChanged(Location loc) {
editLocation.setText("");
pb.setVisibility(View.INVISIBLE);
Toast.makeText(getBaseContext(),"Location changed : Lat: " +
loc.getLatitude()+ " Lng: " + loc.getLongitude(),
Toast.LENGTH_SHORT).show();
String longitude = "Longitude: " +loc.getLongitude();
Log.v(TAG, longitude);
String latitude = "Latitude: " +loc.getLatitude();
Log.v(TAG, latitude);
/*----------to get City-Name from coordinates ------------- */
String cityName=null;
Geocoder geocoder;
List<Address> addresses;
geocoder = new Geocoder(TabClubActivity.this, Locale.getDefault());
try {
addresses = geocoder.getFromLocation(latitude, longitude, 1);
if (!addresses.isEmpty()) {
Address returnedAddress = addresses.get(0);
StringBuilder strReturnedAddress = new StringBuilder("");
for (int i = 0; i < returnedAddress.getMaxAddressLineIndex(); i++) {
strReturnedAddress.append(returnedAddress.getAddressLine(i)).append(" ");
}
strAdd = strReturnedAddress.toString();
textview.setText(strAdd);
Log.e("MyCurrentLoctionAddress", "" + strReturnedAddress.toString());
cityName=addresses.get(0).getLocality();
} else {
// Log.e("MyCurrentLoctionAddress", "No Address returned!");
}
} catch (IOException e) {
e.printStackTrace();
}
String s = longitude+"\n"+latitude +
"\n\nMy Currrent City is: "+cityName;
editLocation.setText(s);
}
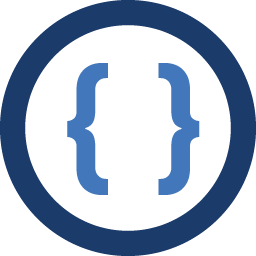
Author by
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I want to create a app to Track the Users who installed my App, So i have a following code for tracking, This code working good but it will return only CITY NAME. But i need full details like street name, city, like wise .
public class GetCurrentLocation extends Activity implements OnClickListener { private LocationManager locationMangaer = null; private LocationListener locationListener = null; private Button btnGetLocation = null; private EditText editLocation = null; private ProgressBar pb = null; private static final String TAG = "Debug"; private Boolean flag = false; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //if you want to lock screen for always Portrait mode setRequestedOrientation(ActivityInfo .SCREEN_ORIENTATION_PORTRAIT); pb = (ProgressBar) findViewById(R.id.progressBar1); pb.setVisibility(View.INVISIBLE); editLocation = (EditText) findViewById(R.id.editTextLocation); btnGetLocation = (Button) findViewById(R.id.btnLocation); btnGetLocation.setOnClickListener(this); locationMangaer = (LocationManager) getSystemService(Context.LOCATION_SERVICE); } @Override public void onClick(View v) { flag = displayGpsStatus(); if (flag) { Log.v(TAG, "onClick"); editLocation.setText("Please!! move your device to" + " see the changes in coordinates." + "\nWait.."); pb.setVisibility(View.VISIBLE); locationListener = new MyLocationListener(); if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) { // TODO: Consider calling // ActivityCompat#requestPermissions // here to request the missing permissions, and then overriding // public void onRequestPermissionsResult(int requestCode, String[] permissions, // int[] grantResults) // to handle the case where the user grants the permission. See the documentation // for ActivityCompat#requestPermissions for more details. return; } locationMangaer.requestLocationUpdates(LocationManager.GPS_PROVIDER, 5000, 10, locationListener); } else { alertbox("Gps Status!!", "Your GPS is: OFF"); } } /*----Method to Check GPS is enable or disable ----- */ private Boolean displayGpsStatus() { ContentResolver contentResolver = getBaseContext() .getContentResolver(); boolean gpsStatus = Settings.Secure .isLocationProviderEnabled(contentResolver, LocationManager.GPS_PROVIDER); if (gpsStatus) { return true; } else { return false; } } /*----------Method to create an AlertBox ------------- */ protected void alertbox(String title, String mymessage) { AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setMessage("Your Device's GPS is Disable") .setCancelable(false) .setTitle("** Gps Status **") .setPositiveButton("Gps On", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // finish the current activity // AlertBoxAdvance.this.finish(); Intent myIntent = new Intent( Settings.ACTION_SECURITY_SETTINGS); startActivity(myIntent); dialog.cancel(); } }) .setNegativeButton("Cancel", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // cancel the dialog box dialog.cancel(); } }); AlertDialog alert = builder.create(); alert.show(); } /*----------Listener class to get coordinates ------------- */ private class MyLocationListener implements LocationListener { @Override public void onLocationChanged(Location loc) { editLocation.setText(""); pb.setVisibility(View.INVISIBLE); Toast.makeText(getBaseContext(),"Location changed : Lat: " + loc.getLatitude()+ " Lng: " + loc.getLongitude(), Toast.LENGTH_SHORT).show(); String longitude = "Longitude: " +loc.getLongitude(); Log.v(TAG, longitude); String latitude = "Latitude: " +loc.getLatitude(); Log.v(TAG, latitude); /*----------to get City-Name from coordinates ------------- */ String cityName=null; Geocoder gcd = new Geocoder(getBaseContext(), Locale.getDefault()); List<Address> addresses; try { addresses = gcd.getFromLocation(loc.getLatitude(), loc .getLongitude(), 1); if (addresses.size() > 0) System.out.println(addresses.get(0).getLocality()); cityName=addresses.get(0).getLocality(); } catch (IOException e) { e.printStackTrace(); } String s = longitude+"\n"+latitude + "\n\nMy Currrent City is: "+cityName; editLocation.setText(s); } @Override public void onProviderDisabled(String provider) { // TODO Auto-generated method stub } @Override public void onProviderEnabled(String provider) { // TODO Auto-generated method stub } @Override public void onStatusChanged(String provider, int status, Bundle extras) { // TODO Auto-generated method stub } } }
-
Steve over 7 years@T.KarthikeyanT.Karthikeyan inside your onlocationchanged use my try and catch block
-
Steve over 7 years@T.KarthikeyanT.Karthikeyan just copy and pate onLocationchanged().I made a change.
-
Admin over 7 yearserror in
addresses = geocoder.getFromLocation(latitude, longitude, 1);
-
Steve over 7 years@T.KarthikeyanT.Karthikeyan you are not getting latitude and longitude. thats why you are getting an error.follow this javapapers.com/android/android-location-fused-provider to get laitude and longitude.
-
Admin over 7 yearsNo, getting latitude and longitude as well as City name
-
Steve over 7 years@T.KarthikeyanT.Karthikeyan what value you are getting in MyCurrentLoctionAddress.debug and tell me
-
Steve over 7 years@T.KarthikeyanT.Karthikeyan put String strAdd = ""; as a global
-
Steve over 7 years@T.KarthikeyanT.Karthikeyan ok
-
Samarth Kejriwal almost 7 yearsI have tried `Geocoder', it won't give me the exact current address of my current location. It gives me an array of addresses of all the nearby locations. I want to get the exact current location. I have my longitude and latitude of the current location.
-
Sriram Nadiminti almost 4 yearsHow to change the frequency of onLocationChange in this case? Searched other messages in the forum, but none of them I could implement.
-
John Melody Me almost 3 yearsVery Helpful. I use your technique to return a string and it works much better